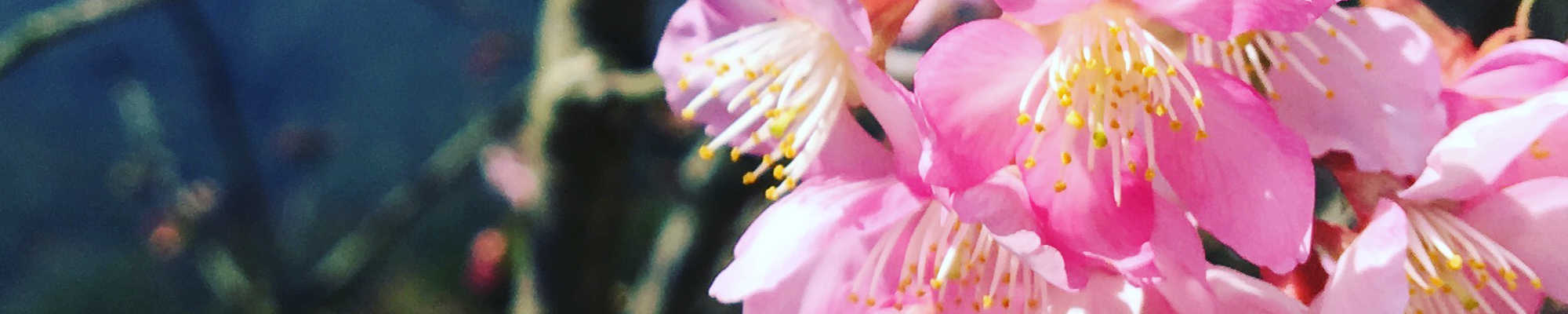
Python 入門指南 5.0
encrypt_view.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 | # 從標準程式庫引入 Tk
from tkinter import *
# 從 tkinter 引入 ttk
from tkinter import ttk
# Encrypt 的 View 類別
class EncryptView(ttk.Frame):
# 建構子設定初值
def __init__(self, master=None):
# 呼叫父類別的建構子
ttk.Frame.__init__(self, master)
# 設定視窗標題
self.winfo_toplevel().title("編密碼小工具")
# 使用格子版面管理員
self.grid()
# 呼叫建立視窗元件的方法
self.createWidgets()
# 建立樣式屬性
self.style = ttk.Style()
# 設定樣式主題
self.style.theme_use("alt")
# 依次取得每一個視窗元件
for child in self.winfo_children():
# 將視窗元件加入邊界
child.grid_configure(padx=5, pady=3)
# 建立所有視窗元件
def createWidgets(self):
# 建立文字輸入標籤
self.input_text = ttk.Label(self)
# 設定文字輸入標籤的文字
self.input_text["text"] = "輸入:"
# 設定文字輸入標籤在格子的位置
self.input_text.grid(row=0, column=0,
sticky=N+E)
# 建立文字輸入欄位
self.input_field = ttk.Entry(self)
# 設定文字輸入欄位的寬度
self.input_field["width"] = 77
# 設定文字輸入欄位在格子的位置
self.input_field.grid(row=0, column=1,
columnspan=6,
sticky=N+W)
# 設定 GUI 開啟後聚焦在文字輸入欄位
self.input_field.focus()
# 設定文字輸出標籤
self.output_text = ttk.Label(self)
# 設定文字輸出標籤的文字
self.output_text["text"] = "輸出:"
# 設定文字輸出標籤格子的位置
self.output_text.grid(row=1, column=0,
sticky=N+E)
# 設定文字輸出欄位
self.output_field = ttk.Entry(self)
# 設定文字輸出欄位的寬度
self.output_field["width"] = 77
# 設定文字輸出欄位在格子的位置
self.output_field.grid(row=1, column=1,
columnspan=6,
sticky=N+W)
# 設定文字輸出欄位無法輸入文字
self.output_field.config(state="disabled")
# 設定新建按鈕在格子的位置
self.new_button = ttk.Button(self)
# 設定新建按鈕的文字
self.new_button["text"] = "新建"
# 設定新建按鈕在格子的位置
self.new_button.grid(row=2, column=0,
sticky=N+W)
# 設定載入按鈕
self.load_button = ttk.Button(self)
# 設定載入按鈕的文字
self.load_button["text"] = "載入"
# 設定載入按鈕在格子的位置
self.load_button.grid(row=2, column=1,
sticky=N+W)
# 設定存檔按鈕
self.save_button = ttk.Button(self)
# 設定存檔按鈕的文字
self.save_button["text"] = "存檔"
# 設定存檔按鈕在格子的位置
self.save_button.grid(row=2, column=2,
sticky=N+W)
# 設定編碼按鈕
self.encode_button = ttk.Button(self)
# 設定編碼按鈕的文字
self.encode_button["text"] = "編碼"
# 設定編碼按鈕在格子的位置
self.encode_button.grid(row=2, column=3,
sticky=N+W)
# 設定解碼按鈕
self.decode_button = ttk.Button(self)
# 設定解碼按鈕的文字
self.decode_button["text"] = "解碼"
# 設定解碼按鈕在格子的位置
self.decode_button.grid(row=2, column=4,
sticky=N+W)
# 設定清除按鈕
self.copy_button = ttk.Button(self)
# 設定清除按鈕的文字
self.copy_button["text"] = "清除"
# 設定清除按鈕在格子的位置
self.copy_button.grid(row=2, column=5,
sticky=N+W)
# 設定拷貝按鈕
self.clear_button = ttk.Button(self)
# 設定拷貝按鈕的文字
self.clear_button["text"] = "拷貝"
# 設定拷貝按鈕在格子的位置
self.clear_button.grid(row=2, column=6,
sticky=N+W)
# 設定訊息欄文字標籤
self.display_text = ttk.Label(self)
# 設定訊息欄文字標籤的文字
self.display_text["text"] = "訊息欄"
# 設定訊息欄文字標籤在格子的位置
self.display_text.grid(row=3, column=0,
columnspan=7,
sticky=N+E)
# GUI 執行部分
if __name__ == '__main__':
# 建立 Tk 應用物件
root = Tk()
# 建立視窗物件
app = EncryptView(master=root)
# 呼叫維持視窗運作的 mainloop()
root.mainloop()
# 檔名: encrypt_view.py
# 說明:《Python入門指南》的範例程式
# 網站: http://kaiching.org
# 作者: 張凱慶
# 時間: 2023 年 6 月
|
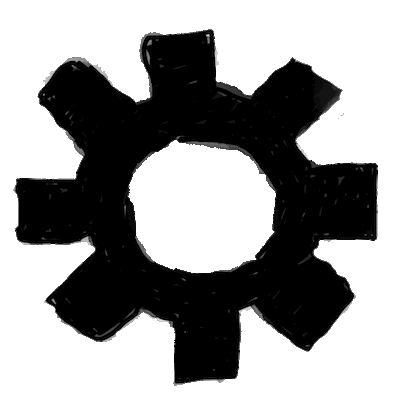