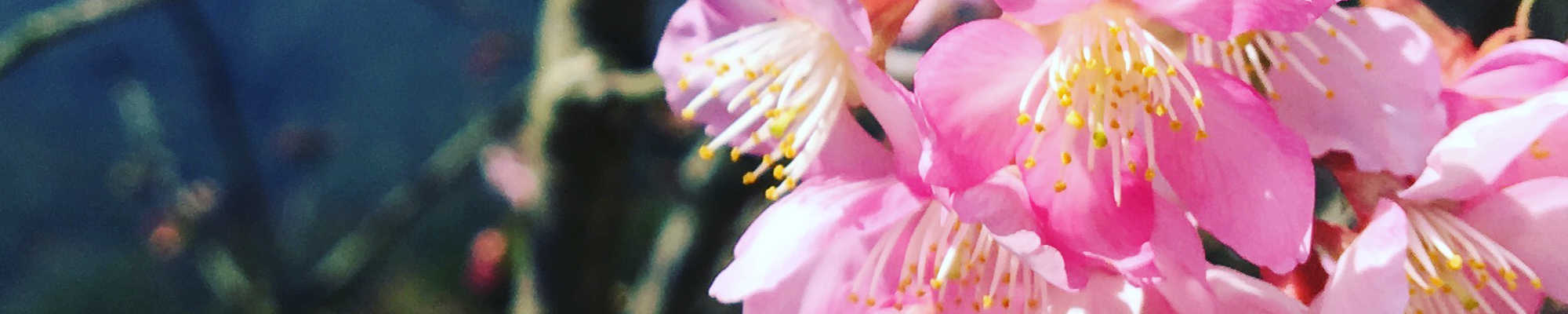
Python 入門指南 5.0
encrypt_controller.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 | # 從標準程式庫 tkinter 引入 Tk
from tkinter import Tk
# 從自定義模組 encrypt 引入 Encrypt
from encrypt import Encrypt
# 從自定義模組 encrypt_view 引入 EncryptView
from encrypt_view import EncryptView
# 從標準程式庫引入 os
import os
# Encrypt 的 Controller 類別
class EncryptController:
# 設定初值
def __init__(self):
# 連結編碼物件的屬性
self.e = None
# 暫存使用者輸入的屬性
self.userinput = ""
# 暫存編碼結果的屬性
self.result = ""
# 設定 EncryptView 屬性
self.app = EncryptView(master=Tk())
# 設定按鈕的事件連結方法
self.app.new_button["command"] = self.new_method
self.app.load_button["command"] = self.load_method
self.app.save_button["command"] = self.save_method
self.app.encode_button["command"] = self.encode_method
self.app.decode_button["command"] = self.decode_method
self.app.clear_button["command"] = self.clear_method
self.app.copy_button["command"] = self.copy_method
# 呼叫維持視窗運作的 mainloop()
self.app.mainloop()
# 按下新建按鈕的事件
def new_method(self):
# 建立新的密碼屬性
self.e = Encrypt()
# 在底下訊息欄顯示密碼表
self.app.display_text["text"] = self.e
# 按下載入按鈕的事件
def load_method(self):
# 先測試檔案是否存在
if os.path.exists("./code.txt"):
# 檔案存在就進行載入工作
with open('./code.txt', 'r') as f:
# 讀取檔案第一行的密碼表
code = f.readline()
# 重新建立密碼屬性
self.e = Encrypt(code)
# 在底下狀態列顯示密碼表
self.app.display_text["text"] = self.e
else:
# 在底下狀態列顯示檔案不存在的提示訊息
self.app.display_text["text"] = "無法載入!"
# 按下儲存按鈕的事件
def save_method(self):
# 先測試是否有按過新建按鈕
if self.e == None:
# 在底下狀態列顯示無法儲存的提示訊息
self.app.display_text["text"] = "無法儲存!"
else:
# 有按過新建按鈕就進行存檔工作
with open('./code.txt', 'w') as f:
# 將密碼表寫入檔案的第一行
f.write(str(self.e)[6:])
# 在底下狀態列顯示工作完成的訊息
self.app.display_text["text"] = "完成存檔!"
# 按下編碼按鈕的事件
def encode_method(self):
# 取得使用者輸入
self.userinput = self.app.input_field.get()
# 先測試使用者是否有輸入
if self.userinput == "":
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = m = "沒有輸入!"
else:
# 再測試是否有按過新建按鈕
if self.e == None:
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = "沒有密碼物件!"
else:
# 使用者有輸入並且有按過新建按鈕
self.result = self.e.toEncode(self.userinput)
# 解鎖輸出欄位
self.app.output_field.config(state="abled")
# 刪除輸出欄位前 200 個字元
self.app.output_field.delete(0, 200)
# 將編碼結果顯示在輸出欄位
self.app.output_field.insert(0, self.result)
# 重新鎖著輸出欄位
self.app.output_field.config(state="disabled")
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = "編碼成功!"
# 按下解碼按鈕的事件
def decode_method(self):
# 取得使用者輸入
self.userinput = self.app.input_field.get()
# 先測試使用者是否有輸入
if self.userinput == "":
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = "沒有輸入!"
else:
# 再測試是否有按過新建按鈕
if self.e == None:
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = "沒有密碼物件!"
else:
# 使用者有輸入並且有按過新建按鈕
self.result = self.e.toDecode(self.userinput)
# 解鎖輸出欄位
self.app.output_field.config(state="abled")
# 刪除輸出欄位前 200 個字元
self.app.output_field.delete(0, 200)
# 將解碼結果顯示在輸出欄位
self.app.output_field.insert(0, self.result)
# 重新鎖著輸出欄位
self.app.output_field.config(state="disabled")
# 在底下狀態列顯示相關訊息
self.app.display_text["text"] = "解碼成功!"
# 按下清除按鈕的事件
def clear_method(self):
# 將密碼屬性設定為 None
self.e = None
# 將暫存使用者輸入的屬性設定為空字串
self.userinput = ""
# 將暫存編碼結果的屬性設定為空字串
self.result = ""
# 刪除輸出入欄位前 200 個字元
self.app.input_field.delete(0, 200)
# 解鎖輸出欄位
self.app.output_field.config(state="abled")
# 刪除輸出欄位前 200 個字元
self.app.output_field.delete(0, 200)
# 重新鎖著輸出欄位
self.app.output_field.config(state="disabled")
# 在底下狀態列顯示清除成功的訊息
self.app.display_text["text"] = "已清除!"
# 按下拷貝按鈕的事件
def copy_method(self):
# 先測試是否有編碼結果
if self.result == "":
# 在底下狀態列顯示拷貝失敗的訊息
self.app.display_text["text"] = "無法拷貝!"
else:
# 清除系統剪貼簿的內容
self.app.clipboard_clear()
# 將結果拷貝到系統剪貼簿
self.app.clipboard_append(self.result)
# 在底下狀態列顯示拷貝成功的訊息
self.app.display_text["text"] = "已拷貝!"
# GUI 執行部分
if __name__ == '__main__':
# 建立 EncryptController 物件
app = EncryptController()
# 檔名: encrypt_controller01.py
# 說明:《Python入門指南》的範例程式
# 網站: http://kaiching.org
# 作者: 張凱慶
# 時間: 2023 年 6 月
|
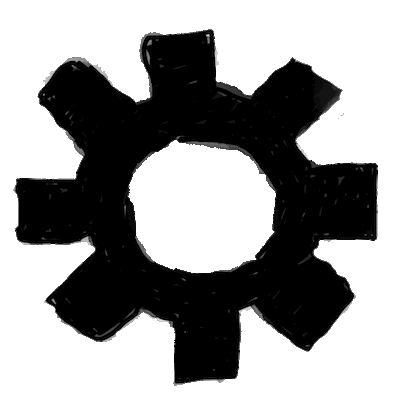