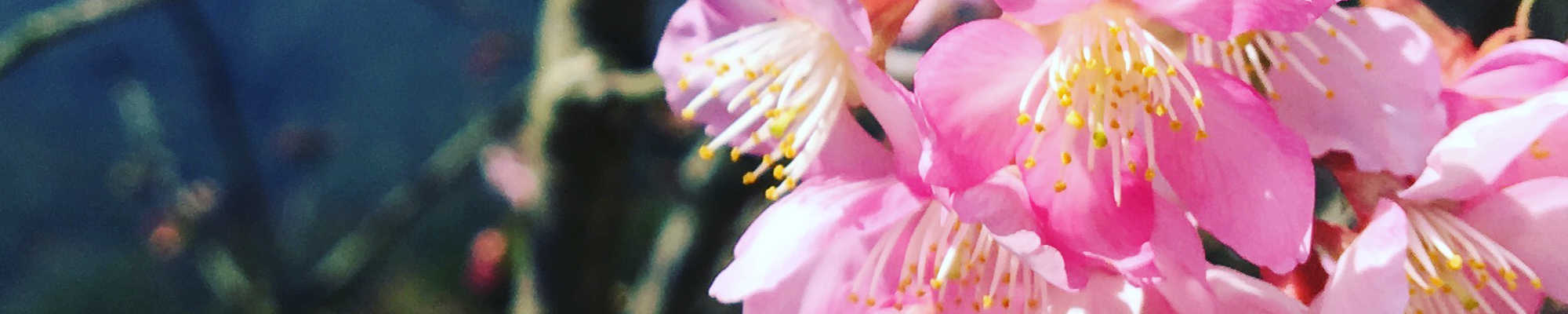
Python 入門指南 5.0
exercise2804.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | # 從 exercise2303 引入 Dice from exercise2303 import Dice # 從 exercise2202 引入 higher from exercise2202 import higher # 從 exercise2802 引入 DiceGame from exercise2802 import examine_int # 從 exercise2201 引入 ParameterError from exercise2201 import ParameterError # 檢查參數是否為字串 def examine_str(p: str) -> bool: try: assert type(p) is str return True except AssertionError: return False # 定義骰子玩家類別 class DiceUser: def __init__(self, name: str, asset: int): # 檢查參數是否正確的型態 if examine_str(name) and examine_int(asset): # 設定玩家名稱 self.name = name # 設定玩家資產 self.asset = asset else: raise ParameterError # 設定骰子 self.dice1 = None self.dice2 = None self.dice3 = None self.dice4 = None # 設定總點數 self.total_points = 0 # 設定擲出骰子數量 self.dice_state = 0 def __str__(self): return self.name # 擲出一顆骰子 def roll1Dice(self): self.dice_state = 1 self.dice1 = Dice() self.total_points = self.dice1.point # 擲出兩顆骰子 def roll2Dice(self): self.dice_state = 2 self.dice1 = Dice() self.dice2 = Dice() self.total_points = self.dice1.point + self.dice2.point # 擲出三顆骰子 def roll3Dice(self): self.dice_state = 3 self.dice1 = Dice() self.dice2 = Dice() self.dice3 = Dice() self.total_points = self.dice1.point + self.dice2.point + self.dice3.point # 擲出四顆骰子 def roll4Dice(self): self.dice_state = 4 self.dice1 = Dice() self.dice2 = Dice() self.dice3 = Dice() self.dice4 = Dice() self.total_points = self.dice1.point + self.dice2.point + self.dice3.point + self.dice4.point # 執行部分 if __name__ == '__main__': try: # 賭注 bet = 0 # 建立莊家 banker = DiceUser("莊家", "500") # 建立玩家並下注 player = DiceUser("玩家", 100) player.asset -= 30 bet += 30 print(player.name + "下注" + str(30)) banker.asset -= 30 bet += 30 # 玩家擲骰子 player.roll2Dice() print(player.name + "擲骰子,擲出 " + str(player.total_points) + " 點") # 莊家擲骰子 banker.roll2Dice() print(banker.name + "擲骰子,擲出 " + str(banker.total_points) + " 點") # 判定輸贏 if banker.total_points >= player.total_points: banker.asset += bet print("莊家贏") else: player.asset += bet print("玩家贏") # 印出玩家最後資產 print(player.name + "有" + str(player.asset)) print(banker.name + "有" + str(banker.asset)) except: print("遊戲建立過程中遭遇問題") # 檔名: exercise2804.py # 說明:《Python入門指南》的練習 # 網站: http://kaiching.org # 作者: 張凱慶 # 時間: 2023 年 11 月 |
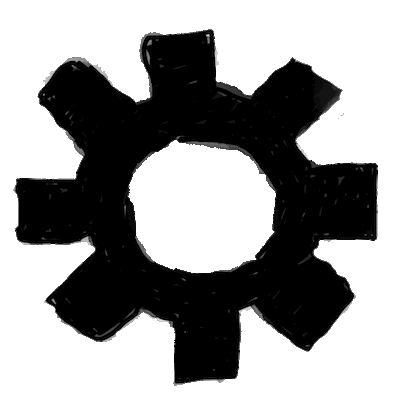