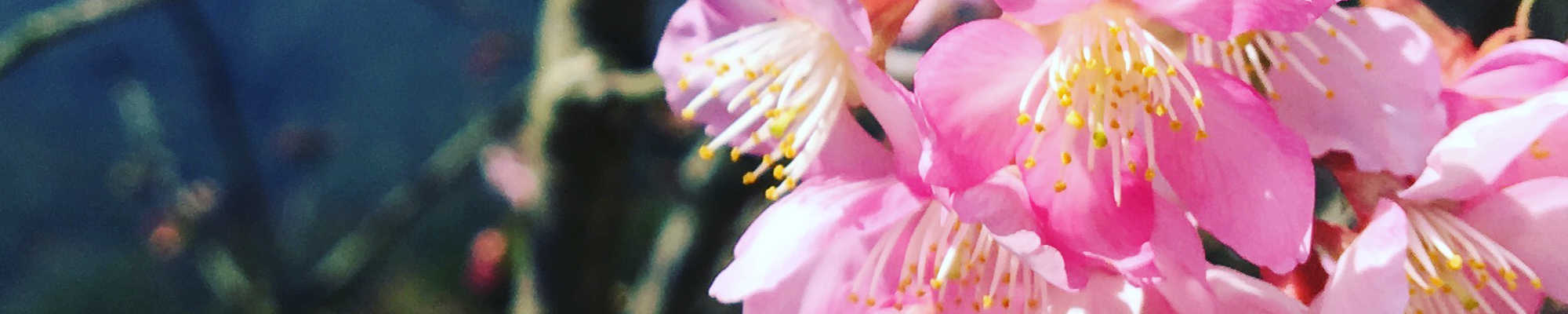
Java 入門指南
單元 23 - 整合 Encrypt 類別
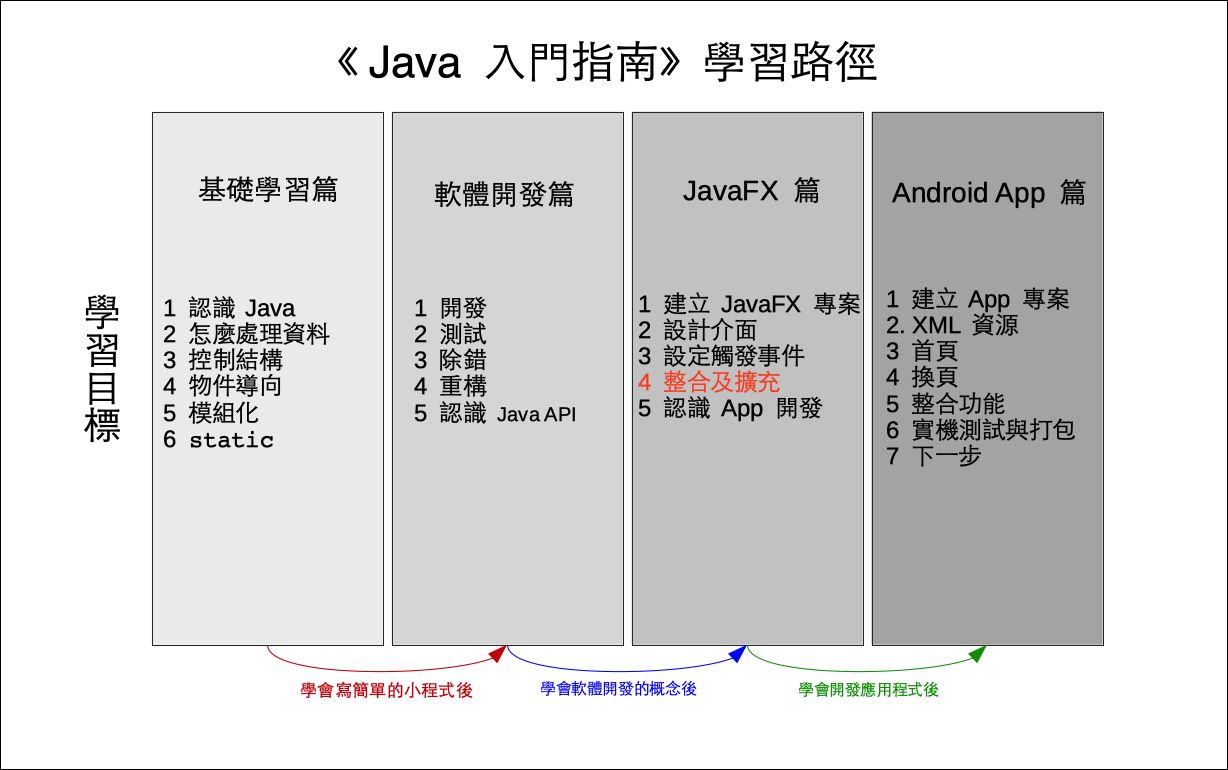
要在 EncryptGUI 專案中整合 Encrypt 類別 (class) ,就是要把定義 Encrypt 類別的 Encrypt.java 拷貝到 EncryptGUI 專案內
首先在專案路徑區 Encrypt 的 Encrypt.java 按滑鼠右鍵,就會出現快顯功能表,這時候點擊 Copy
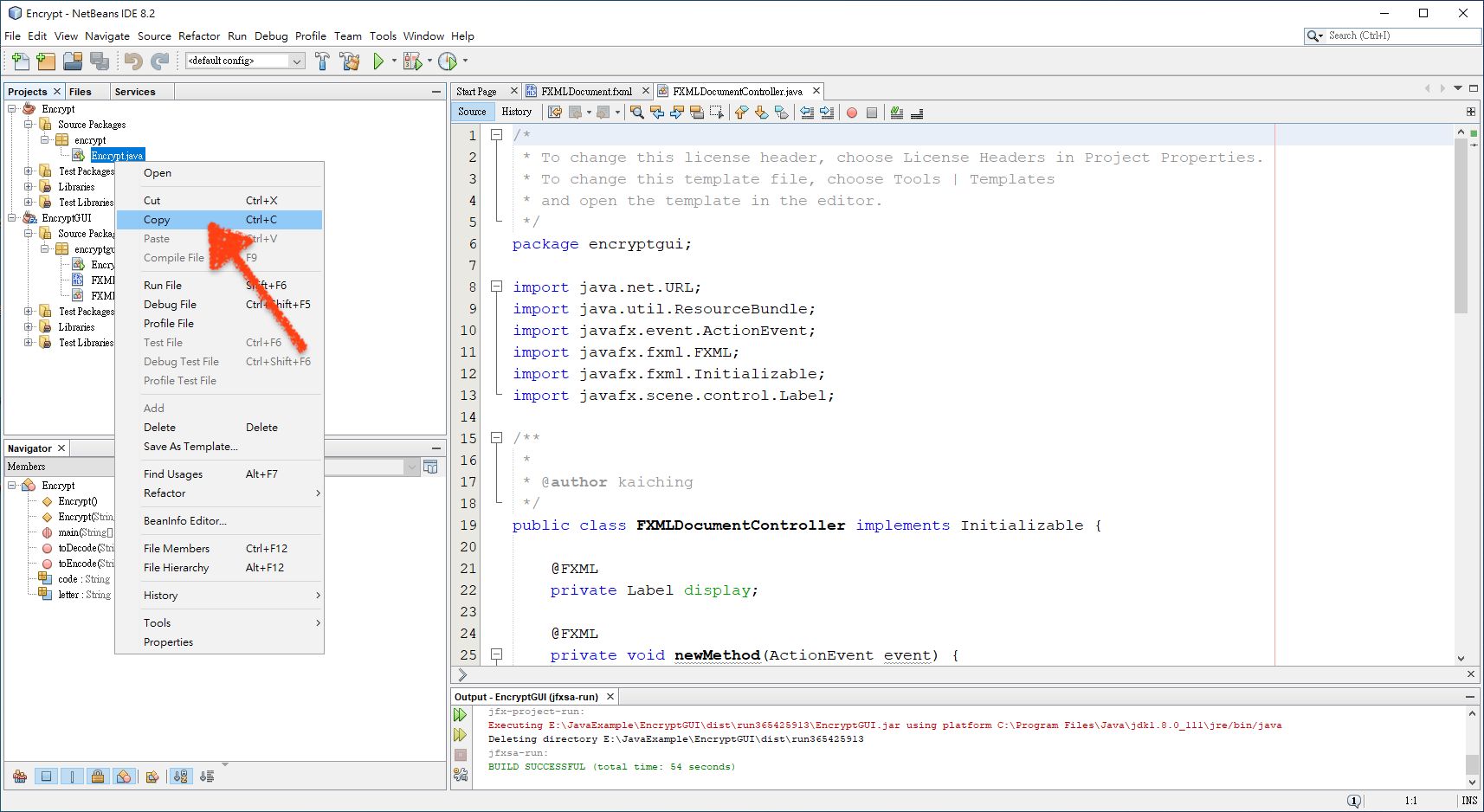
這樣就將 Encrypt.java 複製,然後點選 encryptgui ,按滑鼠右鍵,點擊 Paste 中的 Refactor Paste
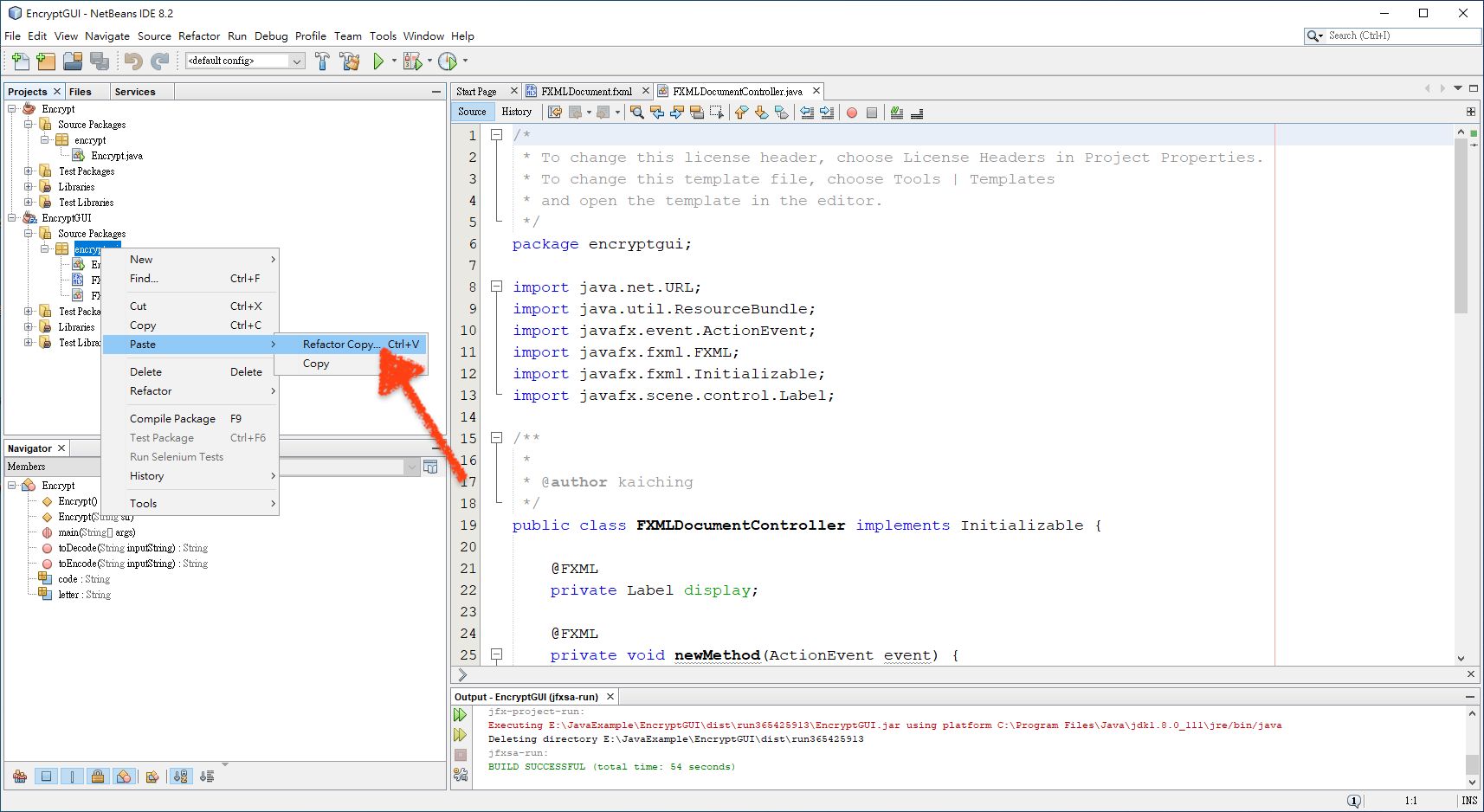
接著出現 Copy Class 視窗,點擊下方的 Refactor 按鈕
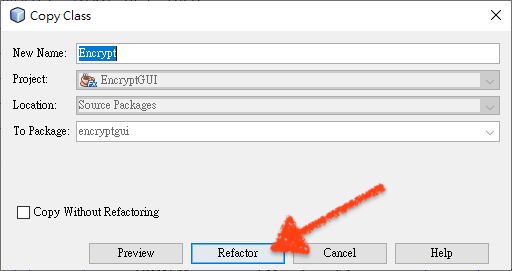
這樣就把 Encrypt.java 複製到 EncryptGUI 專案 (project) 了,利用 Refactor Paste 的原因是 NetBeans 會自動將套件 (package) 改為 encryptgui
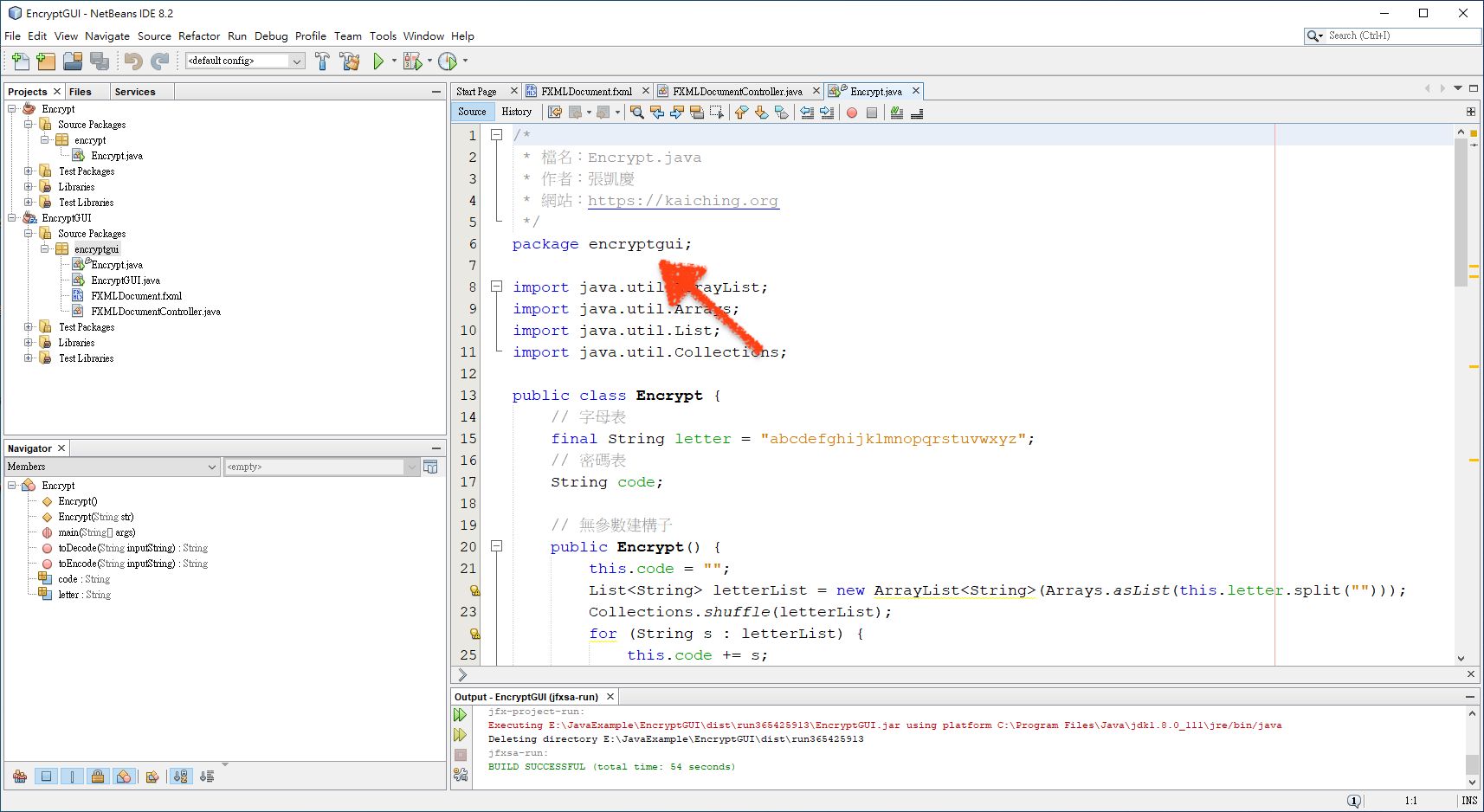
宣告成相同套件就不需要 import ,這是將套件名稱改為 encryptgui 的優點。
然後回到 FXMLDocumentController.java ,增加兩個屬性 (field) ,分別是 Encrypt 型態的 e 與 String 型態的 result
private Encrypt e;
private String result;
完整程式請參考「範例程式篇」的 FXMLDocumentController.java 。
下面繼續增加 @FXML 屬性, TextField 型態的 input 與 ouput
@FXML
private TextField input;
@FXML
private TextField output;
newMethod() 的實作更改如下
@FXML
private void newMethod(ActionEvent event) {
e = new Encrypt();
display.setText(e.code);
}
以上先以關鍵字 (keyword) new 初始化 Encrypt 型態的屬性 e ,然後在 display 顯示密碼表。
如果沒有初始化屬性 e , e 的預設值會是 null 。
編碼方法 (method) encodeMethod() 的實作如下
@FXML
private void encodeMethod(ActionEvent event) {
if (input.getText().isEmpty()) {
display.setText("沒有輸入英文句子!");
}
else {
if (e == null) {
display.setText("沒有密碼物件!");
}
else {
result = e.toEncode(input.getText());
output.setText(result);
display.setText("已編碼");
}
}
}
在 encodeMethod() 方法內用了兩層的 if-else 判斷,首先第一層是利用 input.getText().isEmpty() 判斷 input 是否有內容
if (input.getText().isEmpty()) {
利用 TextField 的 getText() 回傳文字輸入方塊的內容,基本上會是 String 型態的物件,也就是字串 (string) ,再利用字串的 isEmpty() 方法判斷這個字串是不是空字串,如果是空字串,就會回傳 true ,代表使用者沒有輸入,就直接在 display 顯示提示訊息。
如果不用 isEmpty() 方法,可以用 equals() 判斷是否是空字串,這裡需要注意在 Java 中不能直接用兩個等號比較字串的內容是否相等,因為在 Java 中連續兩個等號是比較是否為相同的物件,兩個在不同地方建立的空字串是不同的物件。
反之回傳 false ,表示有輸入,就進入第二層的 if-else
if (e == null) {
display.setText("沒有密碼物件!");
}
else {
result = e.toEncode(input.getText());
output.setText(result);
display.setText("已編碼");
}
第二層的 if 是先判斷屬性 e 是否為 null ,如果屬性 e 是 null ,就表示使用者沒有按過編碼,由於沒有密碼物件,也就無法編碼,因此這裡直接顯示提示訊息。
反之 e 不是 null 就進行編碼工作
result = e.toEncode(input.getText());
output.setText(result);
display.setText("已編碼");
編碼工作就是利用屬性 e 的 toEncode() 方法,然後用 input 的 getText() 取得使用者輸入字串當參數 (parameter) ,再把編碼結果指派給 result 屬性,最後在 display 顯示提示訊息。
解碼方法 decodeMethod() 的實作如下
@FXML
private void decodeMethod(ActionEvent event) {
if (input.getText().isEmpty()) {
display.setText("沒有輸入英文句子!");
}
else {
if (e == null) {
display.setText("沒有密碼物件!");
}
else {
result = e.toDecode(input.getText());
output.setText(result);
display.setText("已解碼");
}
}
}
decodeMethod() 的流程跟 encodeMethod() 一樣,差別在提示訊息跟把呼叫 toEncode() 方法換成 toDecode() 方法。
下面來執行看看囉!先點擊新建按鈕
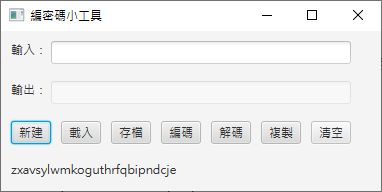
輸入英文句子後,再點擊編碼按鈕
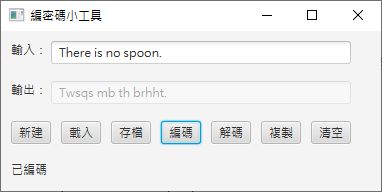
最後將編碼後的文字拷貝到輸入方塊,點擊解碼按鈕
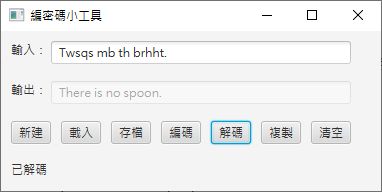
編碼與解碼的功能都正常,下一個單元繼續來實作載入與存檔兩個按鈕。
相關教學影片
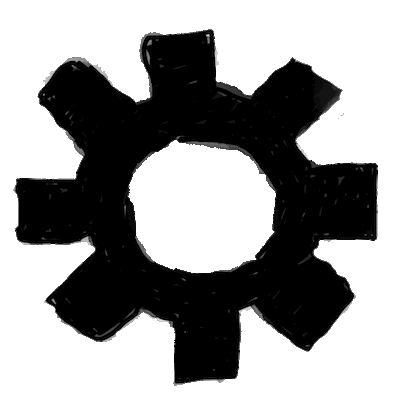
中英文術語對照 | |
---|---|
class | 類別 |
field | 屬性 |
keyword | 關鍵字 |
method | 方法 |
package | 套件 |
parameter | 參數 |
project | 專案 |
string | 字串 |
重點整理 |
---|
1. 將 Encrypt.java 拷貝到 EncryptGUI 專案後,要將 Encrypt 類別的套件名稱改成跟 EncryptGUI 專案一致。 |
2. 在 FXMLDocumentController 類別宣告 Encrypt 型態的屬性 e ,就可以在 FXMLDocumentController 類別中使用 Encrypt 類別的功能。 |
3. 編碼方法跟解碼方法都是先檢查使用者是否有輸入,確認使用者有輸入後,再檢查是否建立 Encrypt 的屬性 e ,雙重確認後才進行編碼或解碼。 |
問題與討論 |
---|
1. 如果 Encrypt.java 拷貝到 EncryptGUI 專案後,不改變套件名稱,那 FXMLDocumentController.java 要怎麼使用 Encrypt 類別呢? |
2. 為什麼編碼或解碼要做雙重檢查?可以不做雙重檢查嗎? |
練習 |
---|
1. 承接上一個單元的 HelloDemo 專案,在 handleButtonAction() 方法中將 count 遞增,這裡可以先暫時在命令列或 label 顯示 count 值。 |
2. 承接上一個單元的 GuessGameDemo 專案,將 Exercise1902 專案的 Guess 類別複製進來。 |
3. 承上題,繼續加入以下四個屬性
|
4. 承上題,新增建構子,並在建構子中將 number 設定為整數 4 。 |