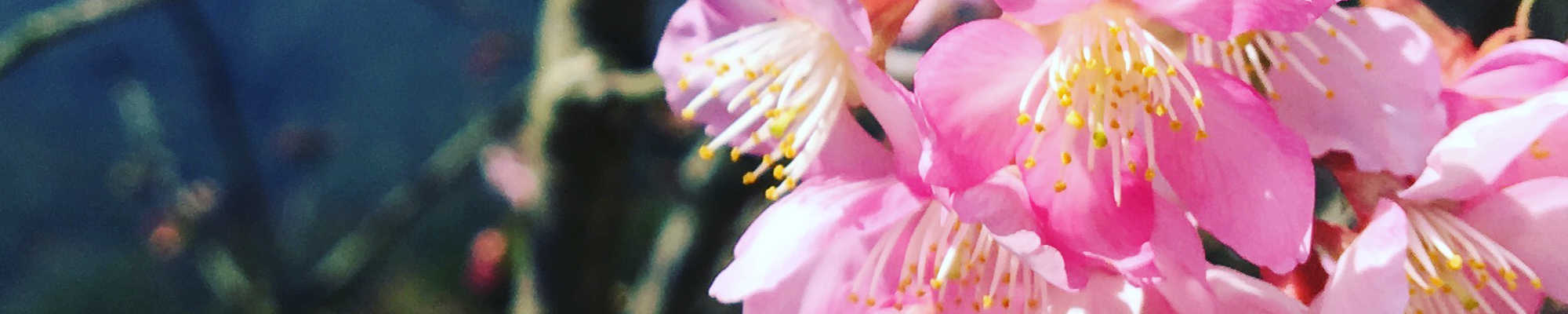
Java 入門指南
單元 12 - static
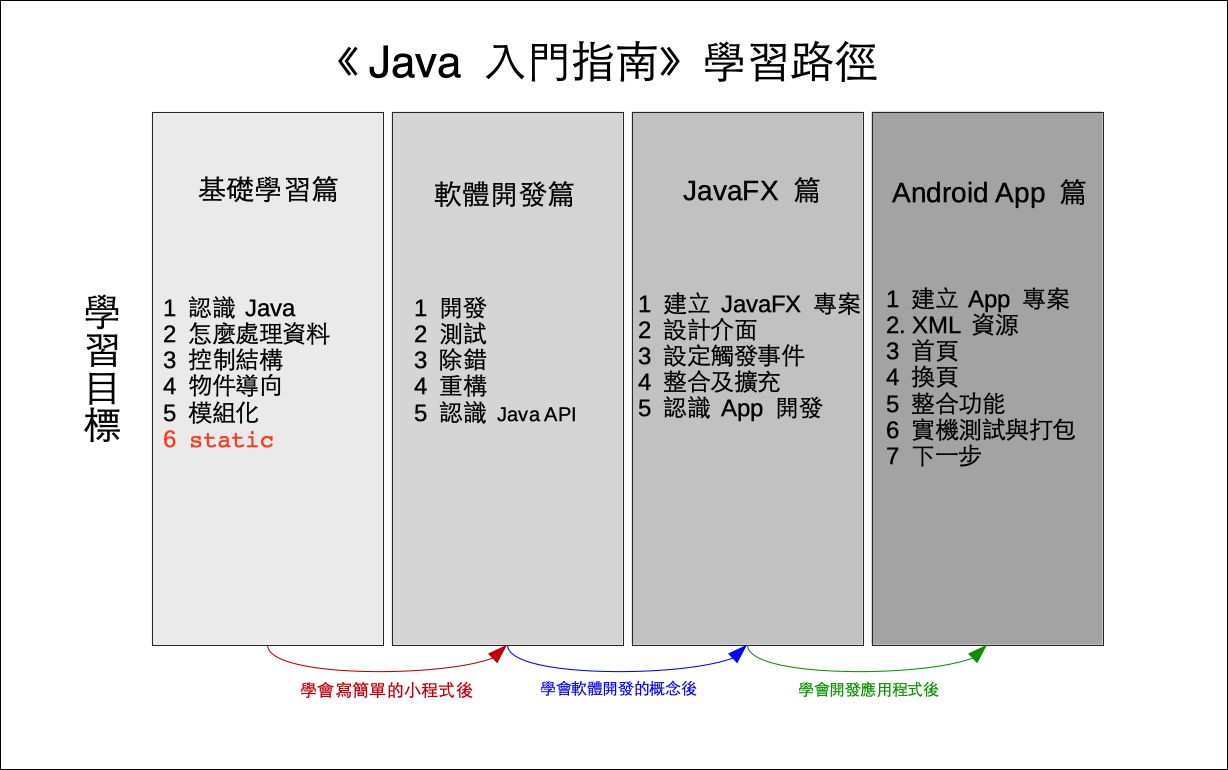
類別 (class) 是物件 (object) 的藍圖,從類別製造出的物件可以有很多個,可是類別仍然只有一個
這是說類別在程式執行時就已存在,如果程式中有用 new 及建構子 (constructor) 建立物件,才會有物件存在,所以物件又被稱為實體 (instance) 。凡是宣告為 static 的屬性與方法都屬於類別,而沒有加上 static 的屬性與方法都屬於物件。
基本上, static 方法 (method) 可以存取 static 屬性 (field) ,若要使用非 static 屬性或呼叫非 static 方法就得建立物件實體,我們不是已經看過很多 static 的 main() 方法例子了嗎?
static 方法也稱為類別方法 (class method) ,同樣 static 屬性也稱為類別屬性 (class attribute) 。
至於存取 static 屬性或 static 方法則用類別名稱即可,舉例如下
/*
* 檔名:StaticDemo.java
* 作者:張凱慶
* 網站:http://kaiching.org
*/
package staticdemo;
public class StaticDemo {
private static int a = 0;
private int b;
public StaticDemo() {
a++;
b = 0;
}
public static double test() {
return ((double) a / 10);
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
StaticDemo d = new StaticDemo();
System.out.println();
int p1 = StaticDemo.a;
System.out.println(p1);
System.out.println(d.b);
double p2 = StaticDemo.test();
System.out.println(p2);
System.out.println();
}
}
}
屬性 a 宣告為 static , static 屬性可以直接指派初值
private static int a = 0;
方法 test() 也宣告為 static
public static double test() {
下方存取 a 或呼叫 test() 都是用類別名稱
int p1 = StaticDemo.a;
System.out.println(p1);
System.out.println(d.b);
double p2 = StaticDemo.test();
System.out.println(p2);
執行結果如下
1 |
0 |
0.1 |
2 |
0 |
0.2 |
3 |
0 |
0.3 |
4 |
0 |
0.4 |
5 |
0 |
0.5 |
6 |
0 |
0.6 |
7 |
0 |
0.7 |
8 |
0 |
0.8 |
9 |
0 |
0.9 |
10 |
0 |
1.0 |
留意 test() 的內容
public static double test() {
return ((double) a / 10);
}
由於 Java 為強型態的程式語言 (programming language) ,所謂強型態除了要宣告 (declare) 型態 (type) 外,計算結果也都得符合所宣告的型態,此例的 test() 需要回傳 double ,這裡的小括弧加上 double 就是強制將 a/10 的計算結果轉換成 double 。
這是因為屬性 a 為 int 型態, int 跟 int 做除法的結果會是 int ,如果沒有進行強制型態轉換的話,迴圈印出的前 9 個值 0/10 為 0 ,後面 1/10 也會是 0 ,要一直到 10/10 等於 1 之後才會是 1 。
int 跟 int 做除法得到 int 是整數除法 (integer division) ,同樣情況也適用在其他整數型態上。
除了除法外,加、減、乘在基本資料型態 (primitive data type) 都會自動進行型態轉換,轉換方向會由儲存範圍小的資料型態轉換成儲存範圍大的資料型態,例如 int 跟 double 相加,編譯器會自動先把 int 轉換成 double ,變成 double 加 double 結果也會是 double 。
若是需要由儲存範圍大的資料型態轉換成儲存範圍小的資料型態,就得進行強制型態轉換,不過這會有遺失精確度 (precision) 的問題。
接下來我們開始著手開發一個編密碼的 Encrypt 類別,繼續介紹更多 Java 程式設計的特性囉!
相關教學影片
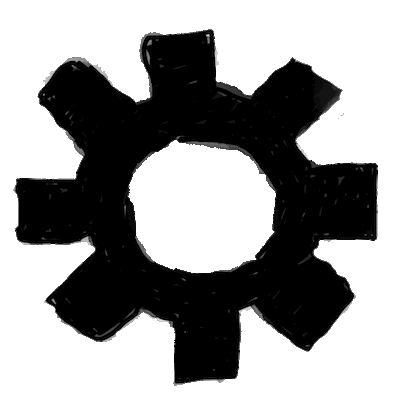
中英文術語對照 | |
---|---|
class | 類別 |
class attribute | 類別屬性 |
class method | 類別方法 |
constructor | 建構子 |
declare | 宣告 |
field | 屬性 |
instance | 實體 |
integer division | 整數除法 |
object | 物件 |
precision | 精確度 |
primitive data type | 基本資料型態 |
programming language | 程式語言 |
method | 方法 |
type | 型態 |
重點整理 |
---|
1. 宣告為 static 的成員屬於類別,由類別物件才能存取。 |
2. static 方法稱為類別方法,同樣 static 屬性也稱為類別屬性。 |
3. 基本資料型態在算術運算會自動進行型態轉換,如果是範圍大的型態轉換成範圍小的型態,可能會有遺失精確度的問題。 |
問題與討論 |
---|
1. 關鍵字 static 的作用為何?方法可以呼叫 static 成員嗎? |
2. 為什麼要分成類別跟實體?兩者有何不同? |
練習 |
---|
1. 建立一個新專案 Exercise1201 ,建立 static 屬性 count ,並直接將 cout 設定為 0 ,然後在建構子中將 count 遞增,執行部分在 main() 中建立 20 個 Exercise1201 物件,最後印出 count 值。 |
2. 承上題,建立一個新專案 Exercise1202 ,設計一個新的 static 的 getCount() 方法用來取得 count 值,執行部分在 main() 中建立 21 個 Exercise1202 物件,最後印出 count 值。。 |