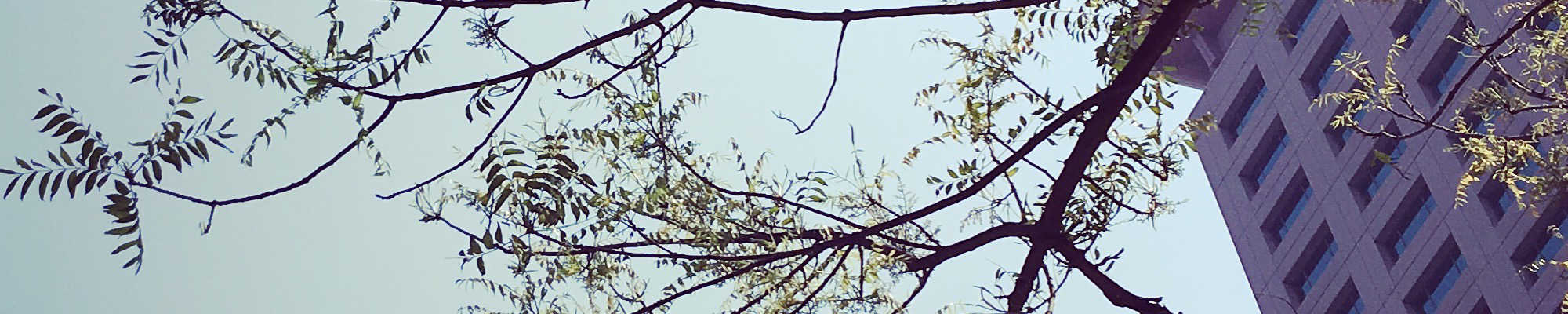
C++ 速查手冊
14.3 - 引入標頭檔
前置處理器指令 #include 用來引入標頭檔 (header file) ,例如引入標準程式庫 (standard library) 的功能
#include <iostream>
#include <string>
int main() {
std::string s = "There is no spoon.";
std::cout << s.find("no") << std::endl;
return 0;
}
/* 《程式語言教學誌》的範例程式
http://kaiching.org/
檔名:u1403_1.cpp
功能:示範 C++ 的前置處理器
作者:張凱慶*/
這裡 iostream 與 string 都是標準程式庫的標頭檔,引入標準程式庫的標頭檔要放在角括號中,也就是大於、小於的符號內
#include <iostream>
#include <string>
由於標準程式庫中的識別字都定義在 std 命名空間中,因此使用標準程式庫的識別字需要加上 std::
std::string s = "There is no spoon.";
可以先用 using namespace std; 省略程式中大量的 std:: 。
string 為標準程式庫中定義的字串物件,這裡使用字串物件的 find() 成員函數找到子字串第一次出現的索引值
std::cout << s.find("no") << std::endl;
編譯執行,結果如下
$ g++ u1403_1.cpp |
$ ./a.out |
9 |
$ |
除了引入標準程式庫的標頭檔外, #include 也用來引入自行定義的標頭檔,通常會把類別、常數、函數原型宣告放在自行定義的標頭檔中,例如我們有以下的標頭檔
class Demo {
public:
int a;
int b;
int do_something();
};
/* 《程式語言教學誌》的範例程式
http://kaiching.org/
檔名:u1403_2.h
功能:示範 C++ 的前置處理器
作者:張凱慶*/
標頭檔為副檔名為 .h 的檔案。
我們用以下的程式引入 u1403_2.h
#include <iostream>
#include "u1403_2.h"
int Demo::do_something() {
return a + b;
}
int main() {
Demo d;
d.a = 1;
d.b = 2;
std::cout << d.do_something() << std::endl;
return 0;
}
/* 《程式語言教學誌》的範例程式
http://kaiching.org/
檔名:u1403_2.cpp
功能:示範 C++ 的前置處理器
作者:張凱慶*/
引入自行定義的標頭檔使用雙引號
#include "u1403_2.h"
實作也會放在獨立的 .cpp 程式檔案
int Demo::do_something() {
return a + b;
}
編譯執行,結果如下
$ g++ u1403_2.cpp |
$ ./a.out |
3 |
$ |
相關教學影片
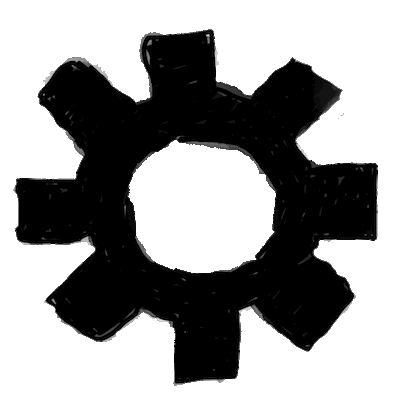