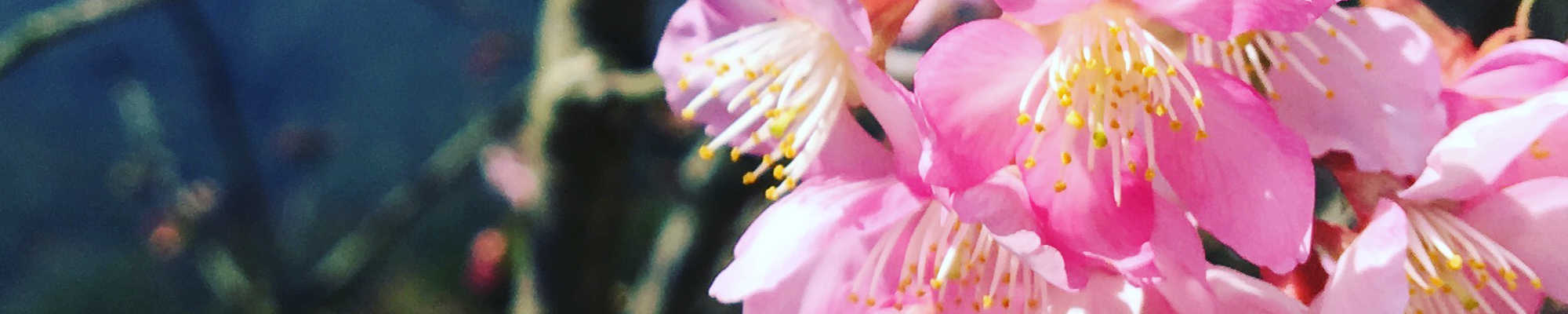
以下為字串的方法列表說明
參數版本 | 功能 |
---|---|
capitalize() | 將字串的首字母改成大寫。 |
casefold() | 回傳 casefold 字串。 |
center(w, f) | 回傳將字串置中的新字串, w 是新字串的總字元數, f 為新增的字元,預設為空格。 |
count(x, i, j) | 回傳字串中 x 的總數,或是在 i 到 j 之間的總數。 |
endswith(s1, s, e) | 判斷字串是否以 s1 結尾,或是從 s 到 e 的範圍以 s1 結尾。 |
expandtabs(tabsize=8) | 回傳以空格數替換字串中的 tab 符號。 |
find(s1, s, e) | 回傳 s1 在字串中的索引值,或是從 s 到 e 範圍中的索引值。 |
format(*a, **k) | 回傳格式化後的新字串。 |
format_map(m) | 利用 m 進行字串格式化。 |
index(s1, s, e) | 回傳 s1 在字串中的索引值,或是從 s 到 e 範圍中的索引值。 |
isalnum() | 判斷字串中所有字元是否都是文字或數字。 |
isalpha() | 判斷字串中所有字元是否都是文字。 |
isdecimal() | 判斷字串中所有字元是否都是十進位數字。 |
isdigit() | 判斷字串中所有字元是否都是數字形式。 |
isidentifier() | 判斷字串是否為內建的識別字,包括關鍵字。 |
islower() | 判斷字串中所有字元是否都是英文小寫字母。 |
isnumeric() | 判斷字串中所有字元是否都是數字化的形式。 |
isprintable() | 判斷字串中所有字元是否都是可印出的形式。 |
isspace() | 判斷字串中所有字元是否為空格。 |
istitle() | 判斷字串是否可作為標題,也就是第一個英文字母是否為大寫。 |
isupper() | 判斷字串中所有字元是否都是英文大寫字母。 |
join(i) | 將字串當連結符號,把迭代器 i 中所有字串元素連結起來。 |
ljust(w, f) | 回傳以 w 為長度,右側以 f 填滿的新字串。 |
lower() | 回傳將英文大寫字母改成英文小寫字母的新字串。 |
lstrip(c) | 回傳去除左側指定 c 字元組合的新字串。 |
str.maketrans(x, y, z) | 回傳 x 與 y 配對的 Unicode 編碼字典,若有提供 z , z 中的字元會跟 None 配對。 |
partition(s) | 回傳以 s 切割成三個子字串的序對。 |
replace(o, n ,c) | 回傳以 n 取代 c 的新字串, c 為替換的數量。 |
rfind(s1, s, e) | 回傳 s1 在字串中最右側的索引值,或是從 s 到 e 範圍中最大的索引值。 |
rindex(s1, s, e) | 回傳 s1 在字串中最右側的索引值,或是從 s 到 e 範圍中最大的索引值。 |
rjust(w, f) | 回傳以 w 為長度,左側以 f 填滿的新字串。 |
rpartition(s) | 回傳從右側以 s 切割成三個子字串的序對。 |
rsplit(sep=None, maxsplit=-1) | 回傳從右側開始以 sep 切割的子字串的串列。 |
rstrip(c) | 回傳去除右側指定 c 字元組合的新字串。 |
split(sep=None, maxsplit=-1) | 回傳以 sep 切割的子字串的串列。 |
splitlines(keepends) | 回傳以 \n 、 \e 、 \f 、 \v 等新行符號切割的子字串的串列。 |
startswith(p, s, e) | 判斷字串是否以 p 開頭,或是從 s 到 e 範圍中以 p 開頭。 |
strip(c) | 回傳將首尾字串組合 c 移除的新字串。 |
swapcase() | 回傳將字串中英文大小寫翻轉的新字串。 |
title() | 回傳作為英文標題的新字串。 |
translate(t) | 回傳以 t 替換的新字串, t 為 maketrans() 回傳的配對物件。 |
upper() | 回傳將英文小寫字母改成英文大寫字母的新字串。 |
zfill(w) | 回傳指定長度 w 並填滿 0 的新字串。 |
str.capitalize()
capitalize() 將字串的首字母改成大寫,舉例如下
print("there".capitalize())
print("hello".capitalize())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr01.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr01.py |
There |
Hello |
$ |
str.casefold()
casefold() 回傳 casefold 字串,因為某些歐洲語言的字母可以轉換成 casefold 形式,也就是可用小寫字母表達的形式,舉例如下
print("groß".casefold())
print("DüRST".casefold())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr02.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr02.py |
gross |
dürst |
$ |
str.center()
center(w, f) 回傳將字串置中的新字串, w 是新字串的總字元數, f 為新增的字元,預設為空格,舉例如下
print("There is no spoon.".center(30))
print("There is no spoon.".center(30, "~"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr03.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr03.py |
There is no spoon. |
~~~~~~There is no spoon.~~~~~~ |
$ |
str.count()
count(x, i, j) 回傳字串中 x 的總數,或是在 i 到 j 之間的總數,舉例如下
a = "吃葡萄吐葡萄皮,不吃葡萄不吐葡萄皮。"
print(a.count("葡萄"))
a = "There is no spoon."
print(a.count("o", 12))
print(a.count("o", 9, 12))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr04.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr04.py |
4 |
2 |
1 |
$ |
str.endswith()
endswith(s1, s, e) 判斷字串是否以 s1 結尾,或是從 s 到 e 的範圍以 s1 結尾,舉例如下
a = "quickly"
print(a.endswith("ly"))
print(a.endswith("ly", 2))
print(a.endswith("ck", 2, 5))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr05.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr05.py |
True |
True |
True |
$ |
str.expandtabs()
expandtabs(tabsize=8) 回傳以空格數替換字串中的 tab 符號,舉例如下
a = "a\t\t\t\ta"
print(a.expandtabs())
print(a.expandtabs(2))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr06.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr06.py |
a a |
a a |
$ |
str.find()
find(s1, s, e) 回傳 s1 在字串中的索引值,或是從 s 到 e 範圍中的索引值,如果沒找到就回傳 -1 ,舉例如下
a = "There is no spoon."
print(a.find("spoon"))
print(a.find("spoon", 1, 10))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr07.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr07.py |
12 |
-1 |
$ |
str.format()
format(*a, **k) 回傳格式化後的新字串,舉例如下
a = 22
b = 99
c = "{0} 加 {1} 等於 {2}"
print(c.format(a, b, a+b))
d = "{name}出生在{country}"
print(d.format(name="他", country="德國"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr08.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr08.py |
22 加 99 等於 121 |
他出生在德國 |
$ |
str.format_map()
format_map(m) 利用 m 進行字串格式化, m 需要是繼承自字典的物件,舉例如下
a = "{name}出生在鄉下"
print(a.format_map(dict(name="他")))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr09.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr09.py |
他出生在鄉下 |
$ |
str.index()
index(s1, s, e) 回傳 s1 在字串中的索引值,或是從 s 到 e 範圍中的索引值,舉例如下,如果沒有找到會發起 ValueError ,舉例如下
a = "There is no spoon."
print(a.index("o"))
print(a.index("o", 12, 16))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr10.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr10.py |
10 |
14 |
$ |
str.isalnum()
isalnum() 判斷字串中所有字元是否都是文字或數字,舉例如下
print("12df".isalnum())
print("你好".isalnum())
print("[][]as".isalnum())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr11.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr11.py |
True |
True |
False |
$ |
str.isalpha()
isalpha() 判斷字串中所有字元是否都是文字,舉例如下
print("12df".isalpha())
print("else".isalpha())
print("你好".isalpha())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr12.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr12.py |
False |
True |
True |
$ |
str.isdecimal()
isdecimal() 判斷字串中所有字元是否都是十進位數字,舉例如下
print("1234".isdecimal())
print("1.34".isdecimal())
print("2e3d".isdecimal())
print("³".isdecimal())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr13.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr13.py |
True |
False |
False |
False |
$ |
str.isdigit()
isdigit() 判斷字串中所有字元是否都是數字形式,舉例如下
print("1234".isdigit())
print("1.34".isdigit())
print("½".isdigit())
print("³".isdigit())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr14.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr14.py |
True |
False |
False |
True |
$ |
str.isidentifier()
isidentifier() 判斷字串是否為內建的識別字,包括關鍵字,舉例如下
print("int".isidentifier())
print("false".isidentifier())
print("for".isidentifier())
print("dict".isidentifier())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr15.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr15.py |
True |
True |
True |
True |
$ |
str.islower()
islower() 判斷字串中所有字元是否都是英文小寫字母,舉例如下
print("1234".islower())
print("abcd".islower())
print("abcD".islower())
print("yes2".islower())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr16.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr16.py |
False |
True |
False |
True |
$ |
str.isnumeric()
isnumeric() 判斷字串中所有字元是否都是數字化的形式,舉例如下
print("1234".isnumeric())
print("1.34".isnumeric())
print("½".isnumeric())
print("³".isnumeric())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr17.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr17.py |
True |
False |
True |
True |
$ |
str.isprintable()
isprintable() 判斷字串中所有字元是否都是可印出的形式,舉例如下
print(" ".isprintable())
print("\a".isprintable())
print("\t".isprintable())
print("abcd".isprintable())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr18.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr18.py |
True |
False |
False |
True |
$ |
str.isspace()
isspace() 判斷字串中所有字元是否為空格,舉例如下
print("\t ".isspace())
print("\t cc".isspace())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr19.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr19.py |
True |
False |
$ |
str.istitle()
istitle() 判斷字串是否可作為標題,也就是第一個英文字母是否為大寫,舉例如下
print("hello".istitle())
print("Hello".istitle())
print("有沒有".istitle())
print("A有沒有".istitle())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr20.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr20.py |
False |
True |
False |
True |
$ |
str.isupper()
isupper() 判斷字串中所有字元是否都是英文大寫字母,舉例如下
print("1234".isupper())
print("ABCD".isupper())
print("ABCd".isupper())
print("YES2".isupper())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr21.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr21.py |
False |
True |
False |
True |
$ |
str.join()
join(i) 將字串當連結符號,把迭代器 i 中所有字串元素連結起來,舉例如下
a = "-"
print(a.join("abcd"))
print(a.join(["1", "2", "3", "4"]))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr22.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr22.py |
a-b-c-d |
1-2-3-4 |
$ |
str.ljust()
ljust(w, f) 回傳以 w 為長度,右側以 f 填滿的新字串, f 預設是空白字元,舉例如下
a = "abcd"
print(a.ljust(12))
print(a.ljust(12, "$"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr23.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr23.py |
abcd |
abcd$$$$$$$$ |
$ |
str.lower()
lower() 回傳將英文大寫字母改成英文小寫字母的新字串,舉例如下
a = "ABCD"
print(a.lower())
a = "HeLLo"
print(a.lower())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr24.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr24.py |
abcd |
hello |
$ |
str.lstrip()
lstrip(c) 回傳去除左側指定 c 字元組合的新字串, c 預設為空白字元,舉例如下
print(" hello ".lstrip())
print("www.example.com".lstrip("w."))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr25.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr25.py |
hello |
example.com |
$ |
str.maketrans()
str.maketrans(x, y, z) 回傳 x 與 y 配對的 Unicode 編碼字典,若有提供 z , z 中的字元會跟 None 配對,舉例如下
print(str.maketrans({}))
print(str.maketrans("abcde", "12345"))
print(str.maketrans("12345", "ABCDE"))
print(str.maketrans("abcde", "fghij", "kmnop"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr26.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr26.py |
{} |
{97: 49, 98: 50, 99: 51, 100: 52, 101: 53} |
{49: 65, 50: 66, 51: 67, 52: 68, 53: 69} |
{112: None, 97: 102, 98: 103, 99: 104, 100: 105, 101: 106, 107: None, 109: None, 110: None, 111: None} |
$ |
str.partition()
partition(s) 回傳以 s 切割成三個子字串的序對,舉例如下
print("There is no spoon.".partition(" "))
print("www.example.com".partition("."))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr27.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr27.py |
('There', ' ', 'is no spoon.') |
('www', '.', 'example.com') |
$ |
str.replace()
replace(o, n ,c) 回傳以 n 取代 c 的新字串, c 為替換的數量,舉例如下
a = "Free your mind."
print(a.replace("mind", "hand"))
a = "What is real? How do you define real?"
print(a.replace("real", "it", 1))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr28.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr28.py |
Free your hand. |
What is it? How do you define real? |
$ |
str.rfind()
rfind(s1, s, e) 回傳 s1 在字串中最右側的索引值,或是從 s 到 e 範圍中最大的索引值,舉例如下
a = "there"
print(a.rfind("e"))
a = "Welcome to the desert... of the real."
print(a.rfind("e", 7, 20))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr29.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr29.py |
4 |
18 |
$ |
str.rindex()
rindex(s1, s, e) ,回傳 s1 在字串中最右側的索引值,或是從 s 到 e 範圍中最大的索引值。如果沒有找到會發起 ValueError ,舉例如下
a = "there"
print(a.rindex("e"))
a = "Welcome to the desert... of the real."
print(a.rindex("e", 7, 20))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr30.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr30.py |
4 |
18 |
$ |
str.rjust()
rjust(w, f) 回傳以 w 為長度,右側以 f 填滿的新字串,舉例如下
a = "abcd"
print(a.rjust(12))
print(a.rjust(12, "$"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr31.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr31.py |
abcd |
$$$$$$$$abcd |
$ |
str.rpartition()
rpartition(s) 回傳從右側以 s 切割成三個子字串的序對,舉例如下
print("There is no spoon.".rpartition(" "))
print("www.example.com".rpartition("."))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr32.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr32.py |
('There is no', ' ', 'spoon.') |
('www.example', '.', 'com') |
$ |
str.rsplit()
rsplit(sep=None, maxsplit=-1) 回傳從右側開始以 sep 切割的子字串的串列,舉例如下
print("Free your mind.".rsplit())
print("There is no spoon".rsplit("n"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr33.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr33.py |
['Free', 'your', 'mind.'] |
['There is ', 'o spoo', ''] |
$ |
str.rstrip()
rstrip(c) 回傳去除右側指定 c 字元組合的新字串, c 預設為空白字元,舉例如下
print(" hello ".rstrip())
print("www.example.com".rstrip(".com"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr34.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr34.py |
hello |
www.example |
$ |
str.split()
split(sep=None, maxsplit=-1) 回傳以 sep 切割的子字串的串列,舉例如下
a = "Free your mind."
b = "Welcome to the desert... of the real."
c = "What is real? How do you define real?"
d = "There is no spoon."
print(a)
print(a.split())
print()
print(b)
print(b.split("o"))
print()
print(c)
print(c.split(" ", 2))
print()
print(d)
print(d.split("a"))
print()
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr43.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr43.py |
Free your mind. |
['Free', 'your', 'mind.'] |
Welcome to the desert... of the real. |
['Welc', 'me t', ' the desert... ', 'f the real.'] |
What is real? How do you define real? |
['What', 'is', 'real? How do you define real?'] |
There is no spoon. |
['There is no spoon.'] |
$ |
str.splitlines()
splitlines(keepends) 回傳以 \n 、 \e 、 \f 、 \v 等新行符號切割的子字串的串列,若將 keepends 設定為 True 就會保留跳脫字元,舉例如下
print("There is no spoon.".splitlines())
print("There\nis\nno\nspoon.\n".splitlines())
print("There\nis\nno\nspoon.\n".splitlines(True))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr35.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr35.py |
['There is no spoon.'] |
['There', 'is', 'no', 'spoon.'] |
['There\n', 'is\n', 'no\n', 'spoon.\n'] |
$ |
str.startswith()
startswith(p, s, e) 判斷字串是否以 p 開頭,或是從 s 到 e 範圍中以 p 開頭,舉例如下
print("prepare".startswith("pre"))
print("prepare".startswith("par", 3, 6))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr36.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr36.py |
True |
True |
$ |
str.strip()
strip(c) 回傳將首尾字串組合 c 移除的新字串,預設移除空白字元,舉例如下
print(" abcd ".strip())
print("www.example.com".strip(".wcom"))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr37.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr37.py |
abcd |
example |
$ |
str.swapcase()
swapcase() 回傳將字串中英文大小寫翻轉的新字串,舉例如下
a = "HeLLo"
a = a.swapcase()
print(a)
a = a.swapcase()
print(a)
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr38.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr38.py |
hEllO |
HeLLo |
$ |
str.title()
title() 回傳作為英文標題的新字串,舉例如下
print("spoon".title())
print("Hello world!".title())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr39.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr39.py |
Spoon |
Hello World! |
$ |
str.translate()
translate(t) 回傳以 t 替換的新字串, t 為 maketrans() 回傳的配對物件,舉例如下
m = str.maketrans("aeiou", "12345")
print("There is no spoon.".translate(m))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr40.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr40.py |
Th2r2 3s n4 sp44n. |
$ |
str.upper()
upper() 回傳將英文小寫字母改成英文大寫字母的新字串,舉例如下
a = "abcd"
print(a.upper())
a = "HeLLo"
print(a.upper())
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr41.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr41.py |
ABCD |
HELLO |
$ |
str.zfill()
zfill(w) 回傳指定長度 w 並填滿 0 的新字串,舉例如下
print("45".zfill(6))
print("-45".zfill(6))
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:tstr42.py
# 功能:示範內建型態
# 作者:張凱慶
於命令列執行以上程式
$ python3 tstr42.py |
000045 |
-00045 |
$ |
相關教學影片
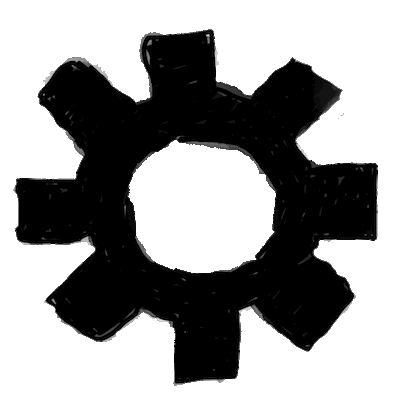