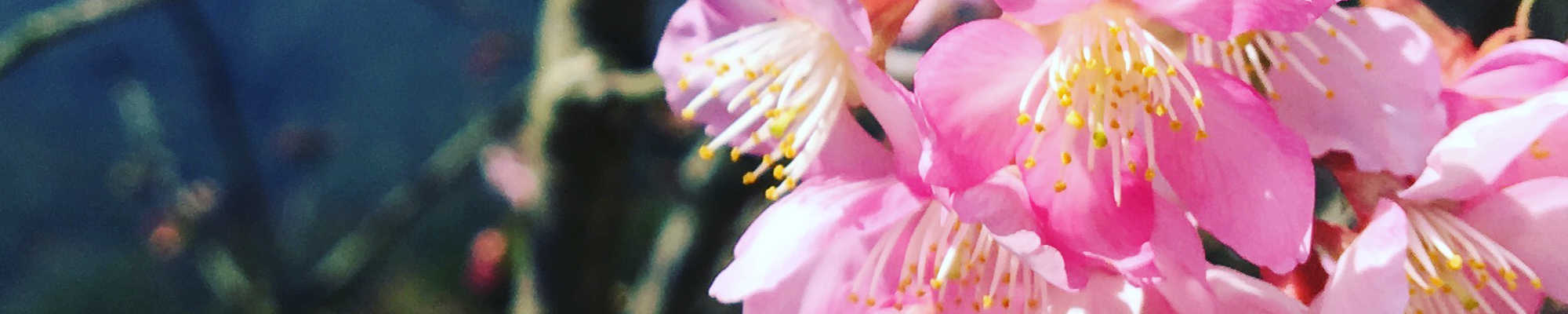
Python 簡易手冊
單元 35 - 格式化字串
格式化字串 (formatted string) 就是讓字串 (string) 依格式可以由變數 (variable) 代入值,現在 Python 的格式化字串分成舊式跟新式兩種,舊式格式化字串是類似 C 語言 printf() 函數 (function) 用的方式,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | # 舊式格式化字串 s = "%(word)s is %(number)d." # 設定要代入的數值 data = {"word": "Three", "number": 3} # 印出舊式格式化字串 print(s % data) # 檔名: string_demo8.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 33 - 字串介紹如何定義字串。
第 2 行,舊式格式化字串是在字串中加上百分比符號,後面用小括弧圍住變數,最後來要加上該變數指定型態的字元,例如 s 是字串, d 則是整數
1 2 | # 舊式格式化字串 s = "%(word)s is %(number)d." |
然後在第 5 行設定 key 為變數字串, value 為代入資料的字典 (dictionary)
4 5 | # 設定要代入的數值 data = {"word": "Three", "number": 3} |
第 8 行印出格式化字串,注意 print() 函數的引數 (argument) 為 s 後面緊接百分比符號,後面再接字典變數
7 8 | # 印出舊式格式化字串 print(s % data) |
s 、 % 與 data 中間有沒有空格都是合法的,此例執行結果如下
> python string_demo8.py |
Three is 3. |
> |
以上可以看到舊式格式化字串還需要指定型態,使用上並沒有很直覺,因此就被新式格式化字串取代,新式格式化字串有兩種,第一種是 f 字串,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | # 設定變數 word = "Three" number = 3 # 設定 f 字串 s = f"{word} is {number}." # 印出 f 字串 print(s) # 檔名: string_demo9.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
第 6 行是 f 字串
5 6 | # 設定 f 字串 s = f"{word} is {number}." |
f 字串中以大括弧圍住變數名稱,變數必須是使用 f 變數前就建立,此例建立在第 2 行及第 3 行
1 2 3 4 | # 設定變數 word = "Three" number = 3 |
如果沒有在使用 f 字串前建立好變數,執行的時候就會報錯,此例執行結果如下
> python string_demo9.py |
Three is 3. |
> |
f 字串中加上格式化的方式是要在變數後加上冒號,然後數字表示該變數總共顯示的位數,字串預設在後面補空格,浮點數變數加上小數點,小數點之前是整數位數,小數點之後是 0 以下的位數,同樣整數前補空格, 0 之後則是補 0 ,浮點數變數的格式化最後要加上 f ,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | # 設定資料字典 menu = {"Burger": 10.0, "Apple": 12.5, "Milk": 8.5, "Sandwich": 14.25} # 用迴圈印出資料字典的內容 for name, price in menu.items(): print(f'{name:10} ==> {price:10.3f}') # 檔名: string_demo10.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
這裡是用字典儲存資料
1 2 3 4 5 | # 設定資料字典 menu = {"Burger": 10.0, "Apple": 12.5, "Milk": 8.5, "Sandwich": 14.25} |
單元 41 - 字典會詳細介紹字典的用法。
底下 for 迴圈 (loop) 是利用字典的 items() 方法 (method) 分別取得字典的 key 及 value
7 8 9 | # 用迴圈印出資料字典的內容 for name, price in menu.items(): print(f'{name:10} ==> {price:10.3f}') |
單元 23 - for 陳述詳細介紹 for 的使用方式。
此例執行結果如下
> python string_demo10.py |
Burger ==> 10.000 Apple ==> 12.500 Milk ==> 8.500 Sandwich ==> 14.250 |
> |
新式格式字串的第二種則是利用字串的 format() 方法,不過只需要使用一般的字串,字串中加入大括弧及索引數,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 | # 設定格式化字串 s = "{0} is no {1}." # 印出格式化字串 print(s.format("There", "spoon")) print(s.format("Here", "sign")) print(s.format("He", "good")) # 檔名: string_demo11.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
格式化字串變數 s 是在第 2 行定義
1 2 | # 設定格式化字串 s = "{0} is no {1}." |
下面 print() 函數是以 s 呼叫 format() 方法,並且提供不同的引數,執行結果如下
> python string_demo11.py |
There is no spoon. Here is no sign. He is no good. |
> |
參考資料
- https://docs.python.org/3/tutorial/inputoutput.html#fancier-output-formatting
- https://docs.python.org/3/reference/lexical_analysis.html#literals
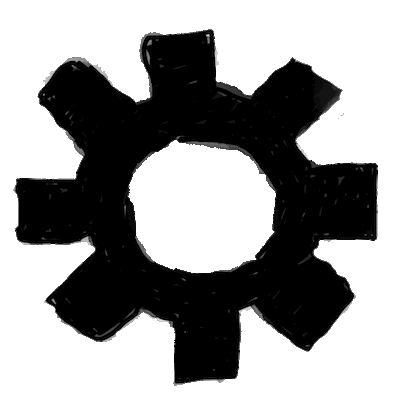