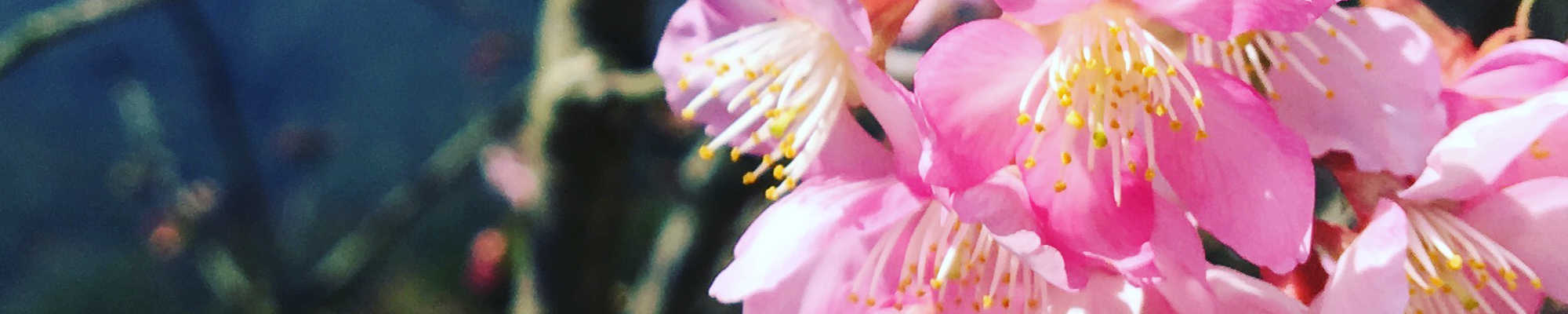
Python 簡易手冊
單元 34 - 字串方法
由於字串 (string) 是很常用的資料型態 (data type) ,因此字串內建很多方法 (method) 可用,底下舉幾個簡單的例子,例如判斷字串是字母、數字或 ASCII 符號
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | # 判斷 1 是字母或數字 print("1".isalnum()) # 判斷 1 是字母 print("1".isalpha()) # 判斷 1 是 ASCII 符號 print("1".isascii()) # 判斷 1 是數字 print("1".isdigit()) # 檔名: string_demo5.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 33 - 字串介紹如何定義字串。
上例每個方法功能如註解 (comment) ,執行結果如下
> python string_demo5.py |
True False True True |
> |
單元 3 - 註解介紹註解的寫法。
以下示範用 find() 尋找子字串的起始索引值, replace() 置換字串內容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | # 建立字串 s = "There is no spoon." # 尋找 spoon 起始字元的索引值 i1 = s.find("spoon") # 建立 spoon 結束字元的索引值 i2 = i1 + len("spoon") # 置換變數 s 的 spoon 為 apple s2 = s.replace("spoon", "apple") # 印出字串 s 及 s2 print(s[i1:i2]) print(s2) # 檔名: string_demo6.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
注意字串是不可變的 (immutable) 資料型態,因此 replace() 會回傳新字串,原字串內容不會有變動,此例執行結果如下
> python string_demo6.py |
spoon There is no apple. |
> |
以下示範用 split() 拆解字串, split() 會依提供的引數 (argument) 字串進行拆解,然後回傳拆解後的字串串列 (list)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | # 建立字串 s = "There is no spoon." # 依空格拆解字串內容,回傳串列 s2 = s.split(" ") # 印出串列中的元素 for i in s2: print(i) # 檔名: string_demo7.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 39 - 串列會詳細介紹串列的用法。
此例執行結果如下
> python string_demo7.py |
There is no spoon. |
> |
參考資料
- https://docs.python.org/3/library/stdtypes.html#text-sequence-type-str
- https://docs.python.org/3/reference/lexical_analysis.html#literals
- https://docs.python.org/3/tutorial/introduction.html#text
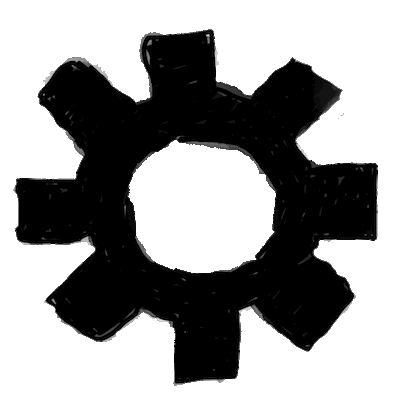