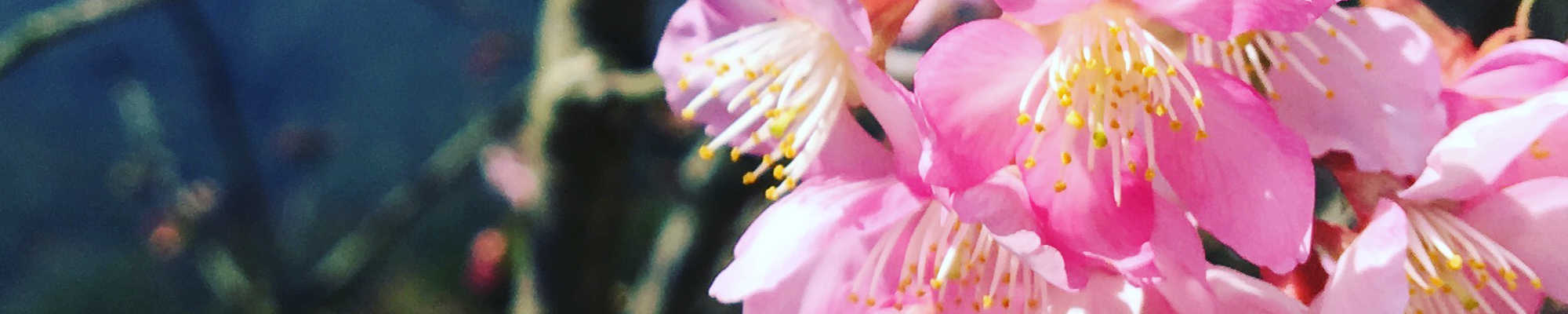
Python 入門指南 5.0
exercise3606.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 | # 從標準程式庫引入 Tk from tkinter import * # 從 tkinter 引入 font from tkinter import font # 從 tkinter 引入 ttk from tkinter import ttk # 引入 Point 定義 from exercise1810 import Point # 引入 Lion 定義 from exercise1812 import Lion # 引入 Elephant 定義 from exercise2901 import Elephant # 引入 Cat 定義 from exercise2902 import Cat # 引入 Mouse 定義 from exercise2903 import Mouse # 引入 move() 定義 from exercise2807 import move # 引入 bound_check() 定義 from exercise2605 import bound_check # 引入 animal_check() 定義 from exercise2809 import animal_check # 引入 get_world_string() 定義 from exercise2906 import get_world_string # 練習用的視窗類別 class JungleGUI(ttk.Frame): def __init__(self, master): ttk.Frame.__init__(self, master) self.winfo_toplevel().title("鬥獸棋") self.root = master self.grid() self.label_font1 = font.Font(master, font="SF 64") self.label_font2 = font.Font(master, font="SF 32") self.style = ttk.Style() self.style.theme_use("alt") self.user = Mouse(Point(3,0), "鼠") self.animals = {} self.animals["鼠"] = self.user self.animals["象"] = Elephant(Point(0,0), "象") self.animals["獅"] = Lion(Point(0,3), "獅") self.animals["貓"] = Cat(Point(3,3), "貓") self.world = [["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"]] self.createWidgets() self.root.bind('<Up>', self.move_up) self.root.bind('<Down>', self.move_down) self.root.bind('<Left>', self.move_left) self.root.bind('<Right>', self.move_right) def createWidgets(self): self.display1 = Label(self) self.display1["text"] = get_world_string(list(self.animals.values()), self.world) self.display1["width"] = 20 self.display1["bg"] = "white" self.display1["fg"] = "black" self.display1["font"] = self.label_font1 self.display1.grid(row=0, column=0, columnspan=3, sticky=N) self.button_up = Button(self) self.button_up["text"] = "上" self.button_up["font"] = self.label_font2 self.button_up["width"] = 5 self.button_up["height"] = 1 self.button_up["command"] = self.move_up self.button_up.grid(row=3, column=1, sticky=N) self.button_down = Button(self) self.button_down["text"] = "下" self.button_down["font"] = self.label_font2 self.button_down["width"] = 5 self.button_down["height"] = 1 self.button_down["command"] = self.move_down self.button_down.grid(row=5, column=1, sticky=N) self.button_left = Button(self) self.button_left["text"] = "左" self.button_left["font"] = self.label_font2 self.button_left["width"] = 5 self.button_left["height"] = 1 self.button_left["command"] = self.move_left self.button_left.grid(row=4, column=0, sticky=N) self.button_right = Button(self) self.button_right["text"] = "右" self.button_right["font"] = self.label_font2 self.button_right["width"] = 5 self.button_right["height"] = 1 self.button_right["command"] = self.move_right self.button_right.grid(row=4, column=2, sticky=N) def move_up(self, event=None): self.animal_move("up") def move_down(self, event=None): self.animal_move("down") def move_left(self, event=None): self.animal_move("left") def move_right(self, event=None): self.animal_move("right") def animal_move(self, d): # 進行移動 if bound_check(self.user, d, self.world): other = animal_check(self.user, list(self.animals.values()), d, self.world) if other == "口": move(self.user, d) else: if other in self.user.food: self.animals.pop(other) move(self.user, d) else: self.animals.pop(self.user.name) # 顯示地圖 self.display1["text"] = get_world_string(list(self.animals.values()), self.world) # 執行部分 if __name__ == '__main__': # 建立 Tk 應用物件 root = Tk() # 建立視窗物件 app = JungleGUI(master=root) # 呼叫維持視窗運作的 mainloop() root.mainloop() # 檔名: exercise3606.py # 說明:《Python入門指南》的練習 # 網站: http://kaiching.org # 作者: 張凱慶 # 時間: 2023 年 12 月 |
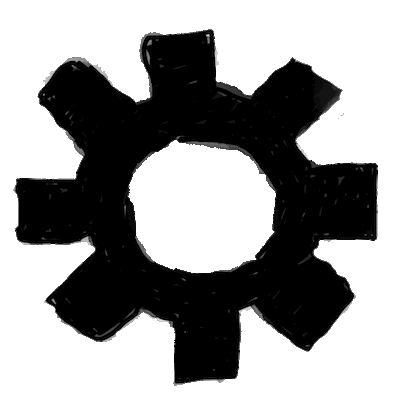