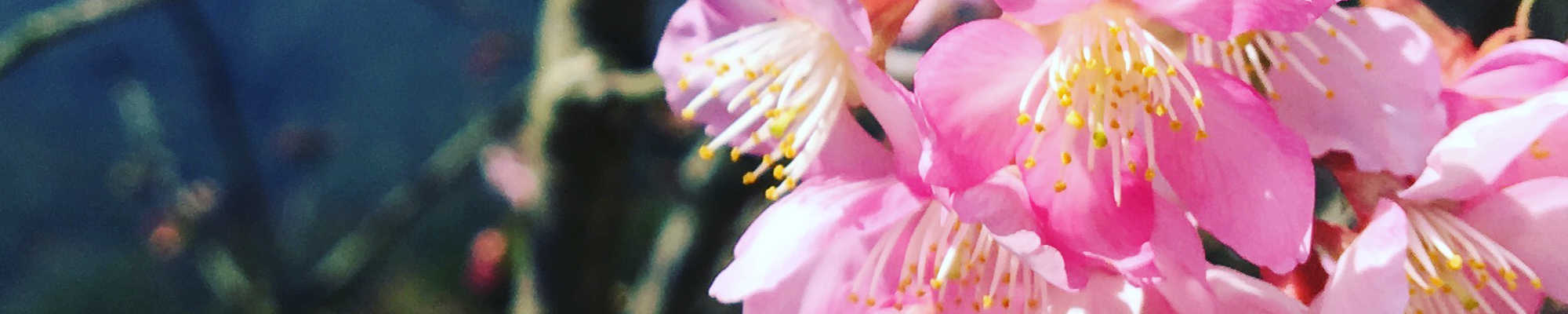
Python 入門指南 5.0
exercise3603.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 | # 引入 Point 定義 from exercise1810 import Point # 引入 Lion 定義 from exercise1812 import Lion # 引入 Elephant 定義 from exercise2901 import Elephant # 引入 Cat 定義 from exercise2902 import Cat # 引入 Mouse 定義 from exercise2903 import Mouse # 引入 examine_animal_list() 定義 from exercise2808 import examine_animal_list # 引入 clear_monitor() 定義 from exercise2604 import clear_monitor # 引入 move() 定義 from exercise2807 import move # 引入 bound_check() 定義 from exercise2605 import bound_check # 引入 animal_check() 定義 from exercise2809 import animal_check # 引入 get_world_string() 定義 from exercise2906 import get_world_string # 引入 time import time # 引入 os import os # 引入 copy import copy # 從 random 引入 randint from random import randint # 從 random 引入 choice from random import choice # 引入 keyboard import keyboard # 產生遊戲結果字串 def generate_result(user, m=None): # 建立動物串列 animals = {"象":Elephant(Point(0,0), "象"), "獅":Lion(Point(0,3), "獅"), "鼠":Mouse(Point(3,0), "鼠"), "貓":Cat(Point(3,3), "貓")} # 設定地圖 world = [["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"]] # 設定移動指令 direction = ["up", "down", "right", "left"] # 移動的迴圈 while len(animals) > 1: # 隨機挑選動物 animal = choice(list(animals.values())) # 狀態變數 state = False # 判斷挑選的動物是否由使用者操控 if animal.name == user: # 印出提示訊息 m("輪到你了~~") # 鍵盤輸入 state = True while state: event = keyboard.read_event() if event.event_type == "down": match event.name: case "down": d = direction[1] case "up": d = direction[0] case "right": d = direction[2] case "left": d = direction[3] break else: m("") d = direction[randint(0, 3)] # 進行移動 if bound_check(animal, d, world): other = animal_check(animal, list(animals.values()), d, world) if other == "口": move(animal, d) else: if other in animal.food: animals.pop(other) move(animal, d) else: animals.pop(animal.name) yield get_world_string(list(animals.values()), world) else: continue # 執行部分 if __name__ == '__main__': # 遊戲模擬迴圈 for game_string in generate_result("鼠", m=print): # 清空螢幕 clear_monitor() # 印出遊戲地圖 print(game_string) # 暫停 0.4 秒 time.sleep(0.4) # 檔名: exercise3603.py # 說明:《Python入門指南》的練習 # 網站: http://kaiching.org # 作者: 張凱慶 # 時間: 2023 年 12 月 |
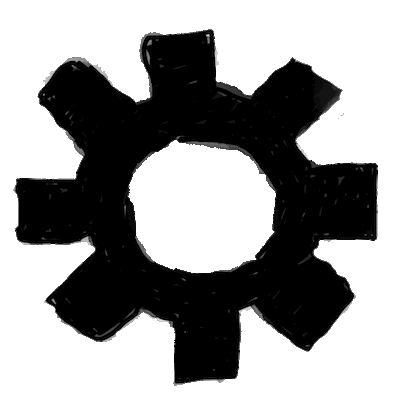