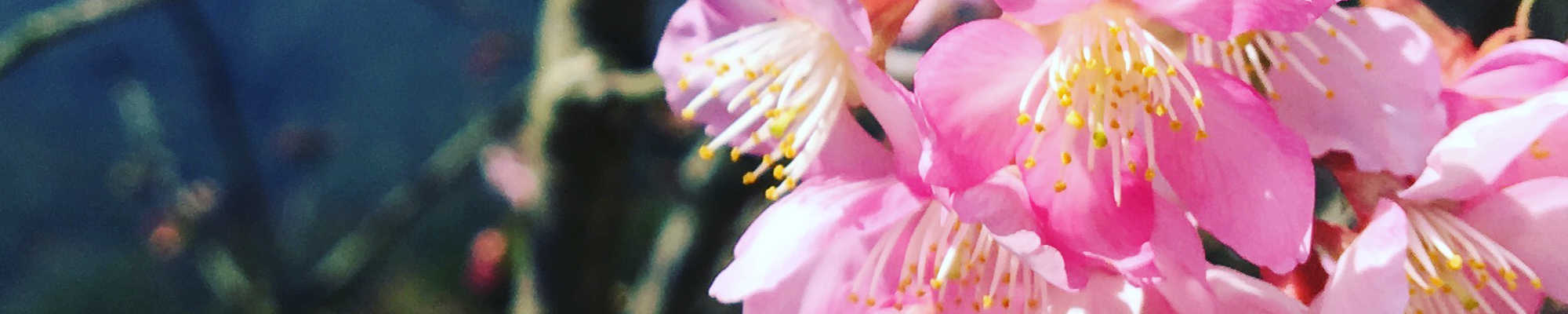
Python 入門指南 5.0
exercise3505.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 | # 從標準程式庫引入 Tk from tkinter import * # 從 tkinter 引入 font from tkinter import font # 從 tkinter 引入 ttk from tkinter import ttk # 從 exercise2705 引入 Guess from exercise2705 import Guess # 練習用的視窗類別 class GuessGUI(ttk.Frame): def __init__(self, master): ttk.Frame.__init__(self, master) self.winfo_toplevel().title("猜數字遊戲") self.root = master self.grid() self.label_font = font.Font(master, font="SF 48") self.createWidgets() self.style = ttk.Style() self.style.theme_use("alt") self.guess = Guess(4) self.count = 0 self.user_input = "" def createWidgets(self): self.display = Label(self) self.display["text"] = "遊戲開始" self.display["width"] = 25 self.display["bg"] = "white" self.display["fg"] = "black" self.display["font"] = self.label_font self.display["anchor"] = "w" self.display.grid(row=0, column=0, columnspan=4, sticky=N) self.button1 = Button(self) self.button1["text"] = "1" self.button1["font"] = self.label_font self.button1["width"] = 5 self.button1["height"] = 1 self.button1.grid(row=1, column=0, sticky=N) self.button2 = Button(self) self.button2["text"] = "2" self.button2["font"] = self.label_font self.button2["width"] = 5 self.button2["height"] = 1 self.button2.grid(row=1, column=1, sticky=N) self.button3 = Button(self) self.button3["text"] = "3" self.button3["font"] = self.label_font self.button3["width"] = 5 self.button3["height"] = 1 self.button3.grid(row=1, column=2, sticky=N) self.button4 = Button(self) self.button4["text"] = "4" self.button4["font"] = self.label_font self.button4["width"] = 5 self.button4["height"] = 1 self.button4.grid(row=1, column=3, sticky=N) self.button5 = Button(self) self.button5["text"] = "5" self.button5["font"] = self.label_font self.button5["width"] = 5 self.button5["height"] = 1 self.button5.grid(row=2, column=0, sticky=N) self.button6 = Button(self) self.button6["text"] = "6" self.button6["font"] = self.label_font self.button6["width"] = 5 self.button6["height"] = 1 self.button6.grid(row=2, column=1, sticky=N) self.button7 = Button(self) self.button7["text"] = "7" self.button7["font"] = self.label_font self.button7["width"] = 5 self.button7["height"] = 1 self.button7.grid(row=2, column=2, sticky=N) self.button8 = Button(self) self.button8["text"] = "8" self.button8["font"] = self.label_font self.button8["width"] = 5 self.button8["height"] = 1 self.button8.grid(row=2, column=3, sticky=N) self.button9 = Button(self) self.button9["text"] = "9" self.button9["font"] = self.label_font self.button9["width"] = 5 self.button9["height"] = 1 self.button9.grid(row=3, column=0, sticky=N) self.button0 = Button(self) self.button0["text"] = "0" self.button0["font"] = self.label_font self.button0["width"] = 5 self.button0["height"] = 1 self.button0.config(state=DISABLED) self.button0.grid(row=3, column=1, sticky=N) self.button_clear = Button(self) self.button_clear["text"] = "清空" self.button_clear["font"] = self.label_font self.button_clear["width"] = 5 self.button_clear["height"] = 1 self.button_clear.grid(row=3, column=2, sticky=N) self.button_again = Button(self) self.button_again["text"] = "再一次" self.button_again["font"] = self.label_font self.button_again["width"] = 5 self.button_again["height"] = 1 self.button_again.config(state=DISABLED) self.button_again.grid(row=3, column=3, sticky=N) # 執行部分 if __name__ == '__main__': # 建立 Tk 應用物件 root = Tk() # 建立視窗物件 app = GuessGUI(master=root) # 呼叫維持視窗運作的 mainloop() root.mainloop() # 檔名: exercise3505.py # 說明:《Python入門指南》的練習 # 網站: http://kaiching.org # 作者: 張凱慶 # 時間: 2023 年 11 月 |
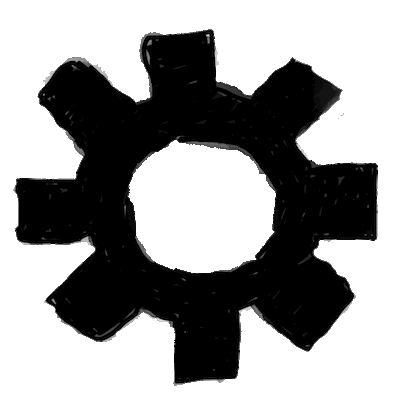