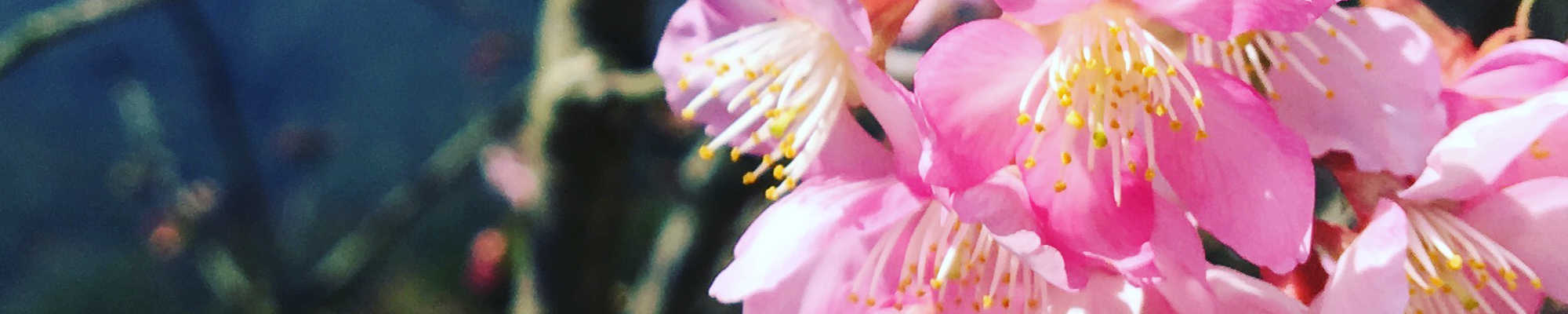
Python 入門指南 5.0
exercise2807.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | # 引入 Point 定義 from exercise1810 import Point # 引入 Animal 定義 from exercise1811 import Animal # 引入 Lion 定義 from exercise1812 import Lion # 引入 print_world() 定義 from exercise2602 import print_world # 引入 clear_monitor() 定義 from exercise2604 import clear_monitor # 引入 examine_str() 定義 from exercise2804 import examine_str # 從 exercise2201 引入 ParameterError from exercise2201 import ParameterError # 引入 time import time # 引入 os import os # 檢查參數是否為 Animal 或其子類別 def examine_animal(p: Animal) -> bool: if issubclass(type(p), Animal): return True else: return False # 改變動物座標 def move(animal: Animal, direction: str): # 檢查參數是否正確的型態 if examine_animal(animal) and examine_str(direction): # 依移動指令移動 match direction: case "up": animal.up() case "down": animal.down() case "right": animal.right() case "left": animal.left() else: raise ParameterError # 執行部分 if __name__ == '__main__': try: # 建立獅子物件 lion = Lion(Point(1,1), "Red") # 設定地圖 world = [["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"], ["口", "口", "口", "口"]] # 清空螢幕 clear_monitor() # 印出地圖 print_world(lion, world) # 設定移動指令 direction = ["right", "down"] # 移動的迴圈 for d in direction: time.sleep(1) clear_monitor() move(lion, d) print_world(lion, world) except: print("遊戲建立過程中遭遇問題") # 檔名: exercise2807.py # 說明:《Python入門指南》的練習 # 網站: http://kaiching.org # 作者: 張凱慶 # 時間: 2023 年 11 月 |
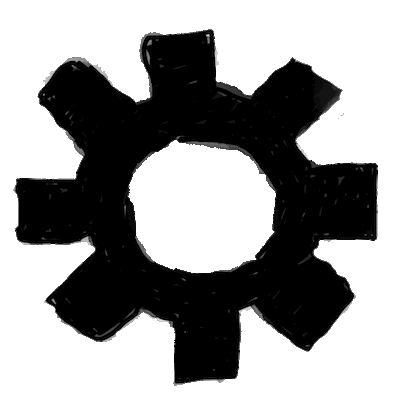