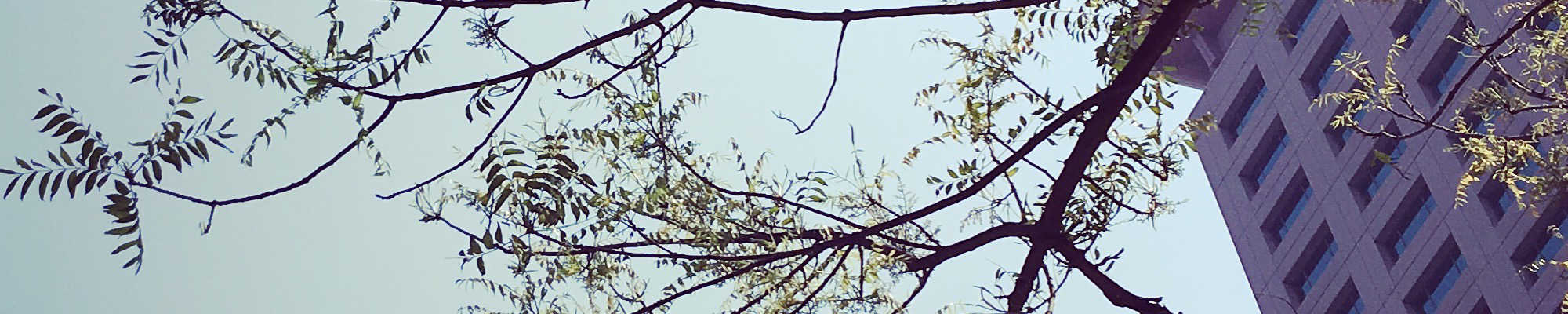
C++ 速查手冊
6.1 - try throw catch 陳述
例外處理為控制程式發生錯誤後的機制, C++ 使用 try 、 throw 與 catch 三個關鍵字 (keyword) 進行例外處理。
try 後面的大括弧用來放可能會發生錯誤的程式碼,在會發生錯誤的地方用 throw 丟出例外 (exception) , catch 依據例外的型態 (type) 進行處理。舉例如下
#include <iostream>
int main() {
int i = -1;
try {
if (i < 0) {
throw "something wrong...";
}
}
catch (const char* message) {
std::cout << message << std::endl;
}
return 0;
}
/* 《程式語言教學誌》的範例程式
http://kaiching.org/
檔名:u0601_1.cpp
功能:示範 C++ 的例外處理
作者:張凱慶*/
假設 i 小於 0 會發生錯誤,因此檢查 i 是否小於 0 ,如果小於 0 就用 throw 丟出 "something wrong..." 的例外
try {
if (i < 0) {
throw "something wrong...";
}
}
對應到 catch 的部份,例外型態就是 const 的字元指標 (pointer)
catch (const char* message) {
std::cout << message << std::endl;
}
意思就是抓到字串 (string) 的例外型態,因為 throw 後面就是丟出字串。
編譯執行,結果如下
$ g++ u0601_1.cpp |
$ ./a.out |
something wrong... |
$ |
例外的型態可以是標準程式庫 (standard library) 中的型態,或是自訂的型態,例如
#include <iostream>
struct BadValue : public std::exception {};
double divide(double a, double b) {
if (b == 0) {
throw BadValue();
}
return a / b;
}
int main() {
try {
std::cout << divide(20, 5) << std::endl;
std::cout << divide(20, 4) << std::endl;
std::cout << divide(20, 3) << std::endl;
std::cout << divide(20, 2) << std::endl;
std::cout << divide(20, 1) << std::endl;
std::cout << divide(20, 0) << std::endl;
}
catch (BadValue e) {
std::cout << "something wrong..." << std::endl;
}
return 0;
}
/* 《程式語言教學誌》的範例程式
http://kaiching.org/
檔名:u0601_2.cpp
功能:示範 C++ 的例外處理
作者:張凱慶*/
這裡的 BadValue 繼承自標準程式庫中的 exception
struct BadValue : public std::exception {};
由於除法在分母為 0 時無法計算,因此在 divide() 函數 (function) 中遇到分母為 0 時就 throw 出一個例外
double divide(double a, double b) {
if (b == 0) {
throw BadValue();
}
return a / b;
}
try 部份的程式碼會逐一執行,碰到發生例外就會跳到 catch 的部份,編譯執行結果如下
$ g++ u0601_2.cpp |
$ ./a.out |
4 |
5 |
6.66667 |
10 |
20 |
something wrong... |
$ |
相關教學影片
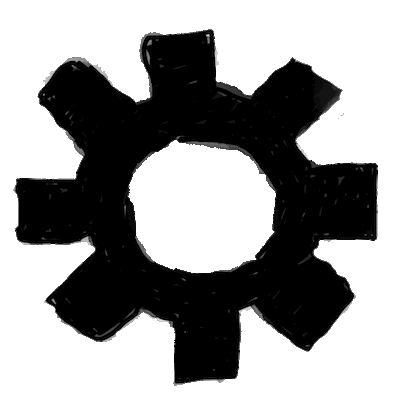