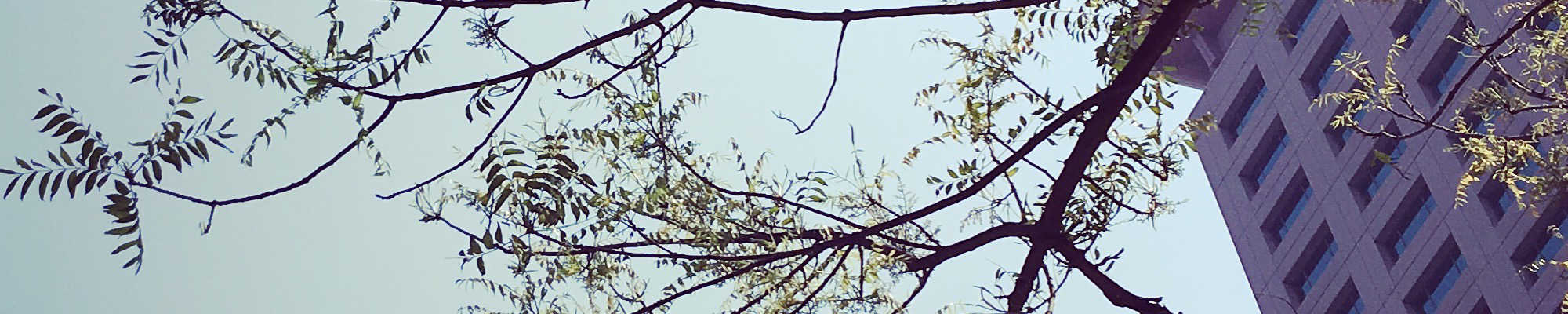
C++ 入門指南 4.01
練習 11.9 參考程式 - 練習替乒乓球類別加入存取函數與修改函數
// 引入標準程式庫中相關的輸入、輸出程式 #include <iostream> // 使用 to_string() #include <string> // std 為標準程式庫的命名空間 using namespace std; // 宣告 Point 類別 class Point { // 宣告 public 成員 public: void set_x(int); void set_y(int); int get_x(); int get_y(); int Distance(Point); private: int x, y; }; // 實作 Point 類別的 setter 成員函數 void Point::set_x(int n) { x = n; } void Point::set_y(int n) { y = n; } // 實作 Point 類別的 getter 成員函數 int Point::get_x() { return x; } int Point::get_y() { return y; } // 宣告 Ball 類別 class Ball { // 宣告 public 成員 public: void set_p(Point); string get_p(); private: Point p; }; // 實作 Ball 類別的 setter 成員函數 void Ball::set_p(Point n) { p.set_x(n.get_x()); p.set_y(n.get_y()); } // 實作 Point 類別的 getter 成員函數 string Ball::get_p() { return "(" + to_string(p.get_x()) + ", " + to_string(p.get_y()) + ")"; } int main(void) { // 宣告建立 Point 物件 Point p; p.set_x(0); p.set_y(0); // 宣告建立 Ball 物件 Ball b; b.set_p(p); // 印出座標 cout << endl; cout << b.get_p() << endl; cout << endl; // 最後回傳 0 給作業系統 return 0; } /* 《程式語言教學誌》的範例程式 http://kaiching.org/ 檔名:exercise1109.cxx 編譯:g++ exercise1109.cxx 執行:./a.out 功能:C++入門指南單元十一的練習 作者:張凱慶 */
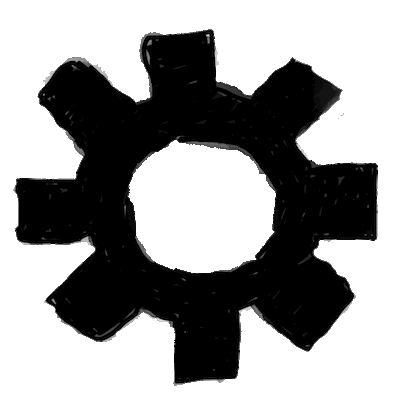