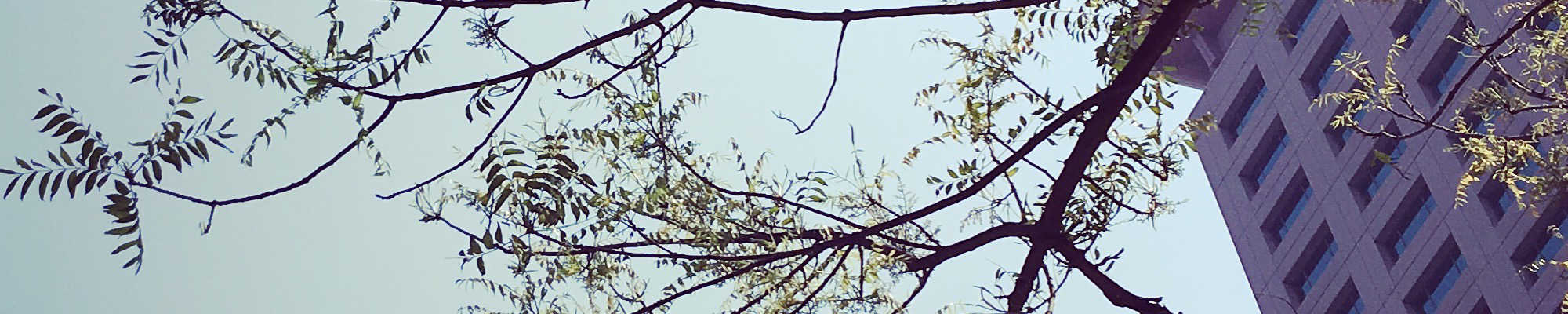
C 速查手冊
11.8 標準程式庫分類索引
- 11.1 數學 math.h
- 11.1.1 double fabs(double);
- 11.1.2 double fmax(double, double);
- 11.1.3 double fmin(double, double);
- 11.1.4 double remainder(double, double);
- 11.1.5 double fma(double, double, double);
- 11.1.6 double round(double);
- 11.1.7 double sqrt(double);
- 11.1.8 double cbrt(double);
- 11.1.9 double pow(double, double);
- 11.1.10 double hypot(double, double);
- 11.1.11 double sin(double);
- 11.1.12 double cos(double);
- 11.1.13 double tan(double);
- 11.1.14 double log(double);
- 11.1.15 double log2(double);
- 11.1.16 double log10(double);
- 11.2 字元測試 ctype.h
- 11.2.1 int isdigit(int);
- 11.2.2 int isalpha(int);
- 11.2.3 int isalnum(int);
- 11.2.4 int isxdigit(int);
- 11.2.5 int islower(int);
- 11.2.6 int isupper(int);
- 11.2.7 int isascii(int);
- 11.2.8 int isblank(int);
- 11.2.9 int isspace(int);
- 11.2.10 int iscntrl(int);
- 11.2.11 int ispunct(int);
- 11.2.12 int isprint(int);
- 11.2.13 int isgraph(int);
- 11.2.14 int tolower(int);
- 11.2.15 int toupper(int);
- 11.3 字串處理 string.h
- 11.3.1 char *strcpy(char *s1, const char *s2);
- 11.3.2 char *strncpy(char *s1, const char *s2, size_t n);
- 11.3.3 char *strcat(char *s1, const char *s2);
- 11.3.4 char *strncat(char *s1, const char *s2, size_t);
- 11.3.5 int strcmp(const char *s1, const char *s2);
- 11.3.6 int strncmp(const char *s1, const char *s2, size_t n);
- 11.3.7 char *strchr(const char *s, int c);
- 11.3.8 size_t strcspn(const char *s1, const char *s2);
- 11.3.9 size_t strspn(const char *s1, const char *s2);
- 11.3.10 char *strpbrk(const char *s1, const char *s2);
- 11.3.11 char *strrchr(const char *s, int c);
- 11.3.12 char *strstr(const char *s1, const char *s2);
- 11.3.13 char *strtok(char *s1, const char *s2);
- 11.3.14 size_t strlen(const char *s);
- 11.3.15 void *memcpy(void *s1, const void *s2, size_t n);
- 11.3.16 void *memmove(void *s1, const void *s2, size_t n);
- 11.3.17 int memcmp(const void *s1, const void *s2, size_t n);
- 11.3.18 void *memchr(const void *s, int c, size_t n);
- 11.3.19 void *memset(void *s, int c, size_t n);
- 11.4 通用工具 stdlib.h
- 11.4.1 double atof(const char *);
- 11.4.2 int atoi(const char *);
- 11.4.3 double strtod(const char *s, char **r);
- 11.4.4 long strtol(const char *s, char **r, int base);
- 11.4.5 void *bsearch(const void *key, const void *base, size_t n, size_t size, int (*cmp)(const void *keyval, const void *datum));
- 11.4.6 void qsort(void *base, size_t n, size_t size, int (*cmp)(const void *, const void *));
- 11.4.7 void *calloc(size_t nobj, size_t size);
- 11.4.8 void *malloc(size_t size);
- 11.4.9 void *realloc(void *ptr, size_t size);
- 11.4.10 void free(void *ptr);
- 11.4.11 void abort(void);
- 11.4.12 void exit(int);
- 11.4.13 int system(const char *s);
- 11.4.14 int rand(void);
- 11.4.15 void srand(unsigned int seed);
- 11.5 時間 time.h
- 11.5.1 clock_t clock(void);
- 11.5.2 time_t time(time_t* timer);
- 11.5.3 double difftime(time_t timer2, time_t timer1);
- 11.5.4 time_t mktime(struct tm* ptm);
- 11.5.5 struct tm* localtime(const time_t* timer);
- 11.5.6 char *asctime(const struct tm* tmptr);
- 11.5.7 char* ctime(const time_t* timer);
- 11.5.8 size t strftime(char* s, size t n, const char* format, const struct tm* tptr);
- 11.6 輸出與輸入 stdio.h
- 11.6.1 int getchar(void);
- 11.6.2 char *gets(char *s);
- 11.6.3 int putchar(int c);
- 11.6.4 int puts(const char *s);
- 11.6.5 int printf(const char *format, ...);
- 11.6.6 int sprintf(char *s, const char *format, ...);
- 11.6.7 int scanf(const char *format, ...);
- 11.6.8 int sscanf(char *s, const char *format, ...);
- 11.7 檔案處理 stdio.h
- 11.7.1 FILE *fopen(const char *filename, const char *mode);
- 11.7.2 FILE *freopen(const char *filename, const char *mode, FILE *stream);
- 11.7.3 int fflush(FILE *stream);
- 11.7.4 int fclose(FILE *stream);
- 11.7.5 int remove(const char *filename);
- 11.7.6 int rename(const char *oldname, const char *newname);
- 11.7.7 int fprintf(FILE *stream, const char *format, ...);
- 11.7.8 int fscanf(FILE *stream, const char *format, ...);
- 11.7.9 int fgetc(FILE *stream);
- 11.7.10 char *fgets(char *s, int n, FILE *stream);
- 11.7.11 int fputc(const char *s, FILE *stream);
- 11.7.12 int fputs(const char *s, FILE *stream);
- 11.7.13 size_t fread(void *ptr, size_t size, siz_t nobj, FILE *stream)
- 11.7.14 size_t fwrite(const void *ptr, size_t size, size_t nobj, FILE *stream);
- 11.7.15 int fseek(FILE *stream, long offset, int origin);
- 11.7.16 long ftell(FILE *stream);
- 11.7.17 void rewind(FILE *stream);
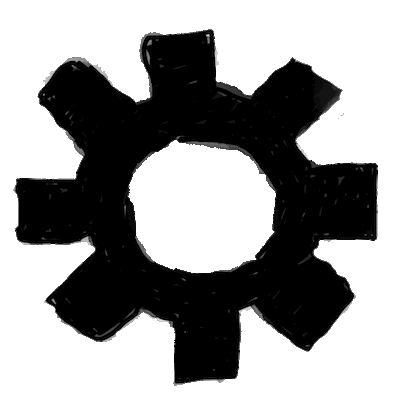