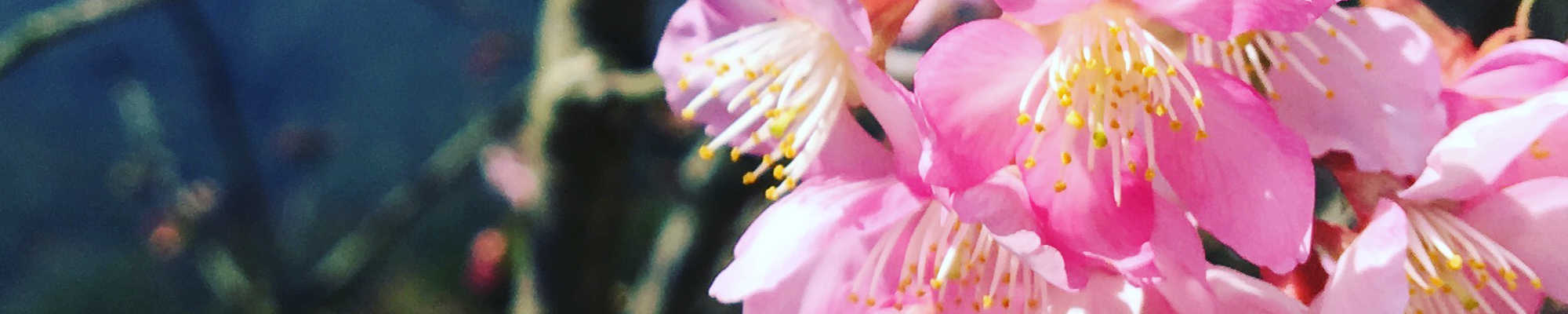
協程函數 (coroutine) 是定義非同步應用程式的語法,所謂非同步應用程式是指程式執行主要任務時會進行等待,等待的同時可以進行其他次要任務,主要任務以及次要任務都完成後,就會結束非同步應用程式。
以下程式示範如何定義協程函數
import asyncio
s = []
async def demo():
i = 0
while i < 10:
print("Quick! You can do what you want to do.")
a = input()
s.append(a)
await asyncio.sleep(2)
print("I can't wait!")
i += 1
asyncio.run(demo())
for i in s:
print(i, end=", ")
#《程式語言教學誌》的範例程式
# http://kaiching.org/
# 檔名:async_demo.py
# 功能:示範非同步應用程式
# 作者:張凱慶
要定義協程函數要先從標準程式庫引入 asyncio ,因為底下要呼叫 sleep() 跟 run() 兩個函數
import asyncio
下面一行定義空串列 s ,這用來在底下暫存資料
s = []
利用關鍵字 async 與 def 宣告定義的函數 demo() 就是協程函數的部分
async def demo(): i = 0 while i < 10: print("Quick! You can do what you want to do.") a = input() s.append(a) await asyncio.sleep(2) print("I can't wait!") i += 1
裡頭用 while 迴圈跑 10 次
while i < 10:
然後印出提示訊息,接收使用者輸入,將使用者輸入的字串加入到 s 中,再來利用關鍵字 await 呼叫 asyncio 中的 sleep() 函數
await asyncio.sleep(2)
sleep() 的參數就是暫停的秒數,最後印出提示訊息,然後控制變數遞增。
下面呼叫 run() ,在 run() 中呼叫 demo() ,最後用 for 迴圈印出串列 s 中的內容
asyncio.run(demo()) for i in s: print(i, end=", ")
換言之,這個程式總共會跑 20 秒,印出第一個提示訊息到第二個提示訊息間隔 2 秒,中間接收使用者輸入,於命令列執行以上程式,會先印出 "Quick! You can do what you want to do."
$ python3 async_demo.py |
Quick! You can do what you want to do. |
等待期間輸入數字 1
1 |
等待 2 秒完成,就會連續印出 "I can't wait!" 及 "Quick! You can do what you want to do."
I can't wait! |
Quick! You can do what you want to do. |
然後依序在等待期間輸入 2 、 3 、 4 、 5 、 6 、 7 、 8 、 9 、 0 ,最後執行結束會先印出 "I can't wait!" ,然後印出所有的數字,如下
I can't wait! |
1, 2, 3, 4, 5, 6, 7, 8, 9, 0, |
相關教學影片
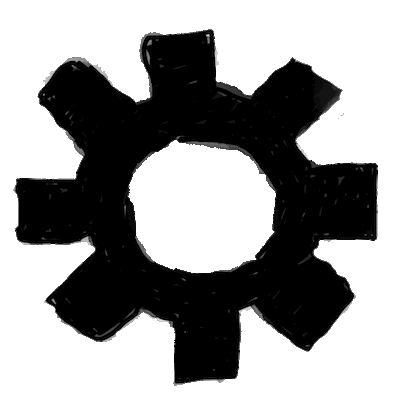