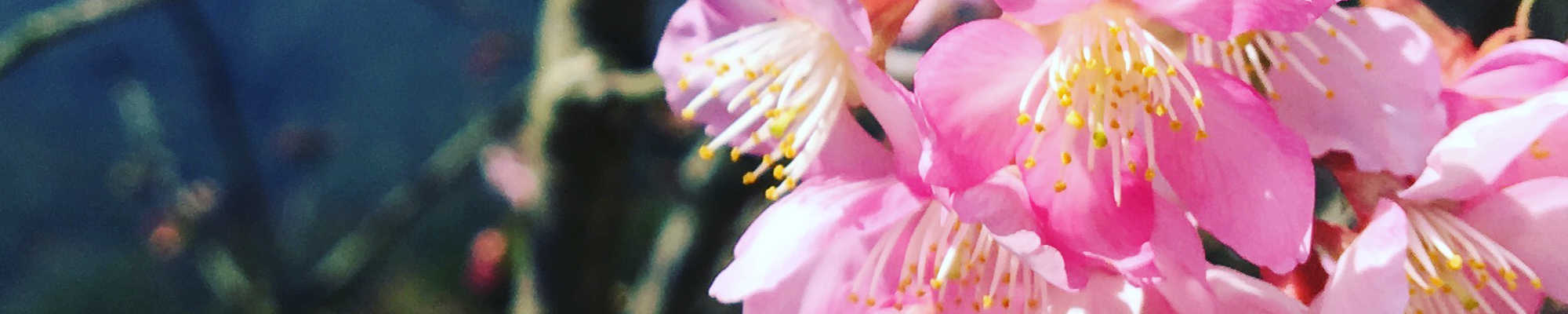
Python 簡易手冊
單元 65 - 抽象方法
抽象方法 (abstract method) 是指在父類別 (superclass) 宣告,然後必須在子類別 (subclass) 實作的方法,這需要用到標準程式庫 (standard library) 中 abc 的 ABC 類別 (class) 與 abstractmethod() 函數 (function) ,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | # 從標準程式庫引入必要內容 from abc import ABC, abstractmethod # 定義繼承自 ABC 的父類別 class Demo(ABC): # 定義抽象方法 @abstractmethod def do_something(self): pass # 定義子類別 class Demo2(Demo): # 實作抽象方法 def do_something(self): print("Something happened...") # 建立子類別物件 d = Demo2() # 呼叫已實作的抽象方法 d.do_something() # 檔名: class_demo30.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 54 - 類別介紹類別的基本概念,單元 62 - 繼承介紹繼承 (inheritance) 的觀念,單元 49 - pass 陳述與省略符號 ...介紹關鍵字 (keyword) pass 的用法,單元 68 - 模組與 import 陳述會介紹 from 與 import 的用法。
第 2 行先引入必要的定義
1 2 | # 從標準程式庫引入必要內容 from abc import ABC, abstractmethod |
父類別 Demo 的 do_something() 的上一行用裝飾子 (decorator) @abstractmethod 宣告,由於要給子類別實作,因此這裡用關鍵字 pass 帶過
4 5 6 7 8 9 | # 定義繼承自 ABC 的父類別 class Demo(ABC): # 定義抽象方法 @abstractmethod def do_something(self): pass |
子類別 Demo2 需要實作抽象方法 do_something() ,這裡簡單印出訊息
11 12 13 14 15 | # 定義子類別 class Demo2(Demo): # 實作抽象方法 def do_something(self): print("Something happened...") |
下面執行部分就是建立 Demo2 物件,然後呼叫 do_something() 方法,執行結果如下
> python class_demo30.py |
Something happened... |
> |
類別方法 (class method) 跟靜態方法 (static method) 一樣可以宣告成抽象方法,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | # 從標準程式庫引入必要內容 from abc import ABC, abstractmethod # 定義繼承自 ABC 的父類別 class Demo(ABC): # 定義抽象類別方法 @classmethod @abstractmethod def do_something(self): pass # 定義抽象靜態方法 @staticmethod @abstractmethod def do_something2(): pass # 定義子類別 class Demo2(Demo): # 實作抽象類別方法 @classmethod def do_something(self): print("Something happened...") # 實作抽象靜態方法 @staticmethod def do_something2(): print("Something happened again...") # 建立物件 d = Demo2() # 呼叫方法 d.do_something() d.do_something2() # 檔名: class_demo31.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 58 - 類別屬性與類別方法介紹如何定義類別方法,單元 59 - 靜態方法介紹如何定義靜態方法。
宣告方式一樣,就是疊了兩個裝飾子,此例執行結果如下
> python class_demo31.py |
Something happened... Something happened again... |
> |
參考資料
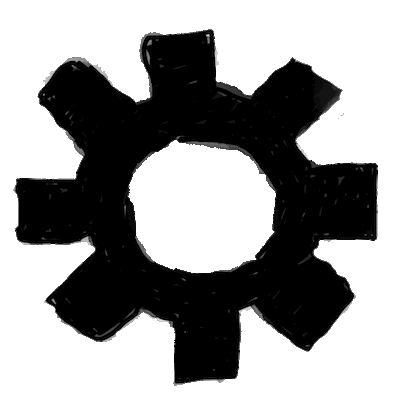