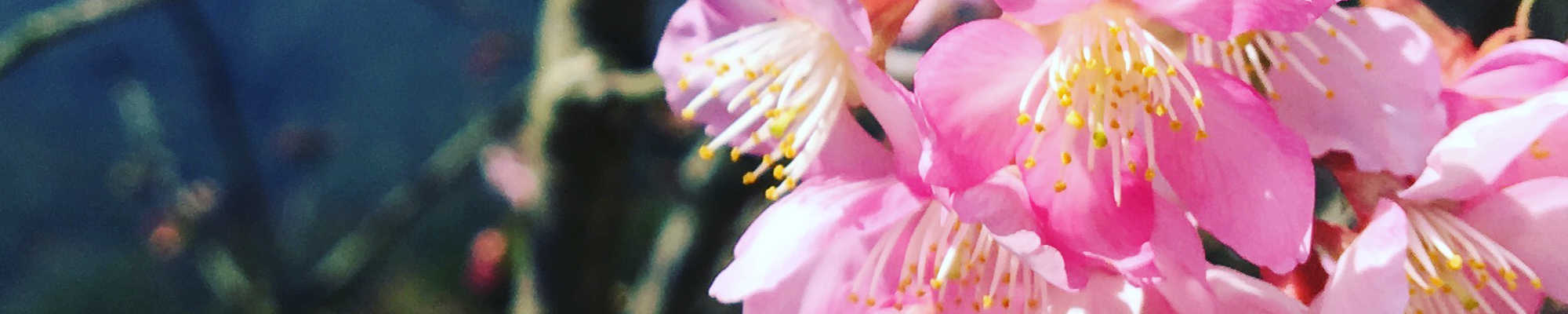
Python 簡易手冊
單元 57 - 字串方法與布林方法
這裡標題講的字串 (string) 方法 (method) 不是字串型態的方法,而是訂製物件資料模型 (data model) 中的 __str__() 、 __repr__() 及 __format__() 方法,這是物件的字串形式,其中如果有設定 __str__() ,那呼叫物件會直接回傳 __str__() 的結果,這也是物件預設的字串形式,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | # 定義類別 class Demo: # 建構子 def __init__(self, p): self.a = p # 物件的字串形式 def __str__(self): return str(self.a) # 印出物件的字串形式 print(Demo("new")) print(Demo(22.22)) print(Demo(22 + 22j)) # 檔名: class_demo7.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
單元 54 - 類別介紹類別 (class) 的基本概念,單元 60 - 資料類別與資料模型會介紹資料模型的基本訂製項目。
第 8 行定義 __str__() 方法,這裡直接回傳 str(self.a) ,也就是實體屬性 (instance attribute) a 的字串形式,此例執行結果如下
> python class_demo7.py |
new 22.22 (22+22j) |
> |
__repr__() 則是在直譯器 (interpreter) 互動介面的字串形式,例如有以下程式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | # 定義類別 class Demo: # 建構子 def __init__(self, p): self.a = p # 物件的字串形式 def __str__(self): return str(self.a) # 互動介面中的字串形式 def __repr__(self): return f"Demo: {str(self.a)}" # 檔名: class_demo8.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
以下示範在互動式介面的顯示結果
>>> from class_demo8 import Demo |
>>> Demo(10) |
Demo: 10 |
>>> |
單元 68 - 模組與 import 陳述會詳細介紹如何使用 from 及 import 陳述 (statement) 。
__format__() 則是可以設定多種格式,使用需要對應到字串的 format() 方法,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | # 資料格式字典 formats = { "normal": "{p.a}", "shell": "Demo: {p.a}" } # 定義類別 class Demo: # 建構子 def __init__(self, p): self.a = p # 在 format() 套用的格式化字串方式 def __format__(self, key): return formats[key].format(p=self) # 印出物件的字串形式 d = Demo(1) print("{:normal}".format(d)) print("{:shell}".format(d)) # 檔名: class_demo9.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
這裡定義兩種格式,分別是 normal 及 shell
1 2 3 4 5 | # 資料格式字典 formats = { "normal": "{p.a}", "shell": "Demo: {p.a}" } |
單元 41 - 字典詳細介紹如何定義字典 (dictionary) 。
第 14 行定義的 __format__() 需要額外定義參數 (parameter) key ,這是資料格式字典中的 key
13 14 15 | # 在 format() 套用的格式化字串方式 def __format__(self, key): return formats[key].format(p=self) |
底下呼叫部分是以格式化字串 (formatted string) 呼叫 format() 方法,並以 Demo 物件當作 format() 的引數 (argument)
17 18 19 20 | # 印出物件的字串形式 d = Demo(1) print("{:normal}".format(d)) print("{:shell}".format(d)) |
此例執行結果如下
> python class_demo9.py |
1 Demo: 1 |
> |
至於布林方法是指定義物件的布林值,這需要定義 __bool__() 方法,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | # 定義類別 class Demo: # 建構子 def __init__(self, p): self.a = p # 物件的布林值 def __bool__(self): if self.a > 10: return True else: return False # 印出物件的布林值 print(bool(Demo(11))) print(bool(Demo(10))) print(bool(Demo(9))) # 檔名: class_demo10.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
這個例子的 Demo 類別依實體屬性 a 來決定真假值, a 大於 10 就是 True ,小於 10 就是 False
7 8 9 10 11 12 | # 物件的布林值 def __bool__(self): if self.a > 10: return True else: return False |
底下分別印出 Demo(11) 、 Demo(10) 與 Demo(9) 的真假值,執行結果如下
> python class_demo10.py |
True False False |
> |
參考資料
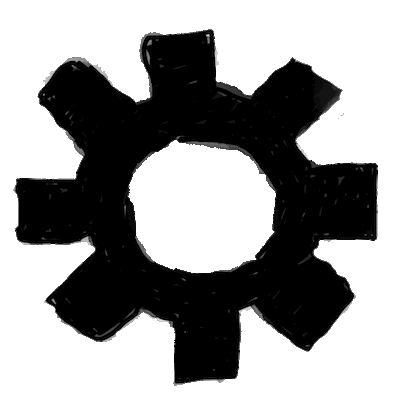