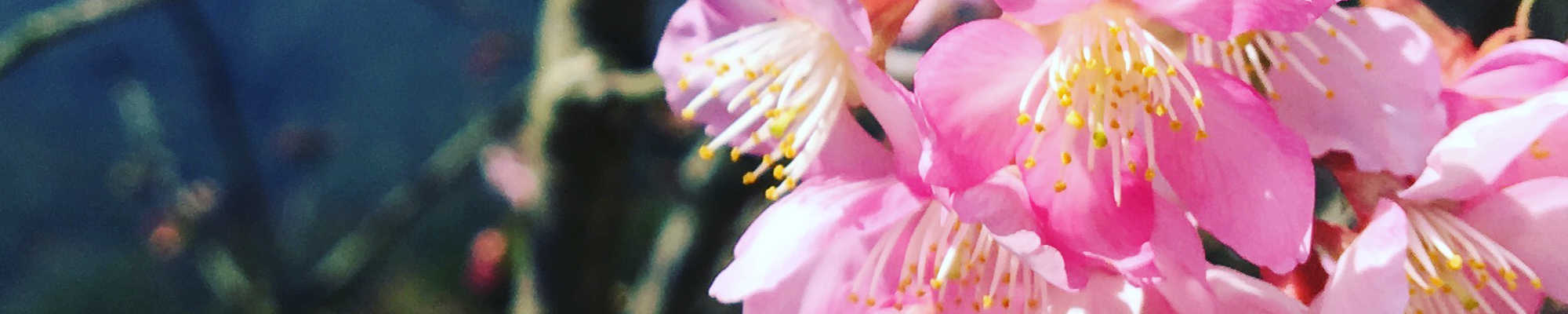
Python 簡易手冊
單元 42 - 集合
Python 中有兩種集合 (set) ,第一種集合是可變的 (mutable) set ,第二種是不可變的 (immutable) frozenset ,以下講集合都是指 set 。集合有以下的操作, s 是集合, x 是元素, iterable 是可迭代的物件
操作 | 說明 |
---|---|
len(s) | 回傳集合 s 的元素總數 |
set([iterable]) | 依 iterable 建立集合物件 |
frozenset([iterable]) | 依 iterable 建立 frozenset 物件 |
x in s | 判斷 x 是否在 s 內 |
x not in s | 判斷 x 是否不在 s 內 |
單元 66 - 迭代器會介紹迭代器 (iterator) 及如何定義迭代器,也會說明可迭代與迭代器的不同。
集合支援以下的運算
運算 | 說明 |
---|---|
s.isdisjoint(other) | 判斷 s 與 other 是否互斥 |
s.issubset(other) | 判斷 s 是否是 other 的子集 |
s >= other | 判斷 s 的元素是否都在 other 中 |
s > other | 判斷 s 的元素是否都在 other 中,但兩者不相等 |
s.issuperset(other) | 判斷 s 是否是 other 的超集 |
s <= other | 判斷 s 的元素是否完全包含 other |
s < other | 判斷 s 的元素是否完全包含 other ,但兩者不相等 |
s.union(*others) | 回傳 s 與 others 的新聯集 |
s | other | ... | 回傳 s 、 other 及更多集合的新聯集 |
s.intersection(*others) | 回傳 s 與 others 的新交集 |
s & other & ... | 回傳 s 、 other 及更多集合的新交集 |
s.difference(*others) | 回傳 s 與 others 的新差集 |
s - other - ... | 回傳 s 、 other 及更多集合的新差集 |
s.symmetric_difference(other) | 回傳 s 與 other 的新對稱差集 |
s ^ other | 回傳 s 與 other 的新對稱差集 |
s.copy() | 回傳 s 的淺層拷貝 |
s.update(*others) | 將 others 的元素加入 s 中 |
s |= other | ... | 將 other 及更多集合的元素加入 s 中 |
s.intersection_update(*others) | 保留 s 中 others 的元素 |
s &= other & ... | 保留 s 中 other 及更多集合的元素 |
s.difference_update(*others) | 保留 s 中不存在於 others 的元素 |
s -= other | ... | 保留 s 中不存在於 other 及更多集合的元素 |
s.symmetric_difference_update(other) | 保留 s 與 other 非共有的元素 |
s ^= other | 保留 s 與 other 非共有的元素 |
s.add(elem) | 在 s 中加入新元素 elem |
s.remove(elem) | 移除 s 中的元素 elem ,如不存在會發起例外 |
s.discard(elem) | 移除 s 中如果存在的元素 elem |
s.pop() | 移除 s 中的任意元素,如果 s 是空集合會發起例外 |
s.clear() | 清空 s 中的所有元素 |
*other 的 other 之前加上星號 * ,這是不限個數引數 (arbitrary argument lists) ,在單元 47 - 不限個數引數會介紹如何定義。
集合的字面常數 (literal) 為大括弧,如下例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | # 以字面常數定義集合 a = {1, 1, 2, 2, 3} print(a) # 進行元素判斷 print(1 in a) print(2 not in a) # 集合運算 print(a - {2, 5, 6}) print(a | {1, 3, 5, 7, 9}) print(a & {1, 3, 5, 7, 9}) print(a ^ {1, 3, 5, 7, 9}) print(a >= {1, 3, 5, 7, 9}) print(a <= {1, 3, 5, 7, 9}) # 檔名: set_demo.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
上例主要是用集合的字面常數建立集合,然後用集合相關的運算子 (operator) 進行計算,執行結果如下
> python set_demo.py |
{1, 2, 3} True False {1, 3} {1, 2, 3, 5, 7, 9} {1, 3} {2, 5, 7, 9} False False |
> |
以下是用內建函數 (built-in function) set() 建立集合
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | # 以字串建立集合 a = set("12345") print(a) print(len(a)) # 以串列建立集合 b = set([1, 2, 3, 4, 5]) print(b) # 檔名: set_demo2.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
這裡字串 (string) 是會切割成單一字元為集合的元素 (element) ,至於串列 (list) 元素就會是集合的元素,此例執行結果如下
> python set_demo2.py |
{'4', '2', '3', '1', '5'} 5 {1, 2, 3, 4, 5} |
> |
以下示範集合的方法 (method)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | # 建立集合 a = {1, 2, 3, 4, 5} b = {1, 3, 5} # 呼叫方法 print(a.isdisjoint(b)) print(a.issubset(b)) print(a.issuperset(b)) print(a.union(b)) print(a.intersection(b)) print(a.difference(b)) print(a.symmetric_difference(b)) print(a.copy()) # 檔名: set_demo3.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
執行結果如下
> python set_demo3.py |
False False True {1, 2, 3, 4, 5} {1, 3, 5} {2, 4} {2, 4} {1, 2, 3, 4, 5} |
> |
參考資料
- https://docs.python.org/3/tutorial/datastructures.html#sets
- https://docs.python.org/3/library/stdtypes.html#set-types-set-frozenset
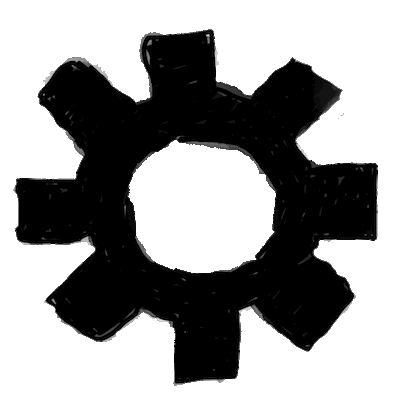