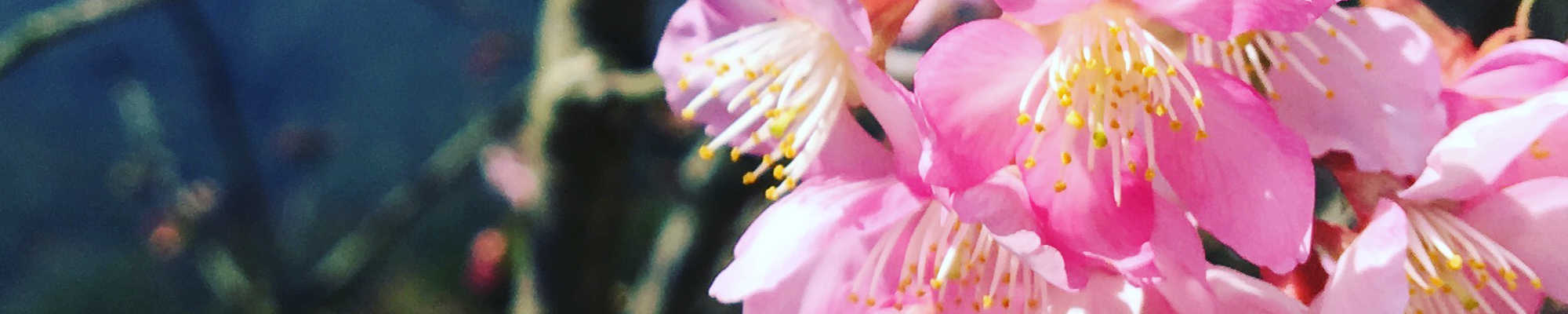
Python 簡易手冊
單元 31 - type 陳述
type 原本是內建函數 (built-in function) ,後來在 Python 3.12 新增 type 為特定場合使用的軟關鍵字 (soft keyword) ,主要用途是定義標準程式庫 (standard library) typing 中的 TypeAlias 型態,這是對原本型態名稱定義別名,舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | # 從標準程式庫 typing 引入 TypeAlias from typing import TypeAlias # 設定 int 別名 Integer1 = int # 型態標記設定 int 別名 Integer2: TypeAlias = int # 以 type 設定 int 別名 type Integer3 = int # 印出型態名稱 print(type(Integer1)) print(type(Integer2)) print(type(Integer3)) # 檔名: type_demo.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
第 5 行,這裡是把 int 指派給 Integer1 ,這樣的話, Integer1 就會是 int ,兩者是一樣的
4 5 | # 設定 int 別名 Integer1 = int |
第 8 行,雖然 Integer2 標記為 TypeAlias ,但是一樣是直接把 int 指派給 Integer2 ,這樣的話, Integer2 一樣會是 int
7 8 | # 設定 int 別名 Integer1 = int |
第 11 行,利用關鍵字 type 設定 Integer3 為 int ,這樣 Integer3 會是 TypeAlias
10 11 | # 以 type 設定 int 別名 type Integer3 = int |
底下印出 Integer1 、 Integer2 及 Integer3 的型態名稱,執行結果如下
> python type_demo.py |
<class 'type'> <class 'type'> <class 'typing.TypeAliasType'> |
> |
型態識別字 (identifier) 的型態名稱就是 'type' ,而關鍵字 type 定義的會是 typing 的 TypeAliasType 。
關鍵字 type 定義的型態可以在型態標記上提供彈性,例如可以使用 | 運算子 (operator) 讓一種型態別名囊括數種型態,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | # 設定 Number 為整數或浮點數 type Number = int | float # 計算總和的函數 def mysum(*p: Number) -> Number: result = 0 for i in p: result += i return result # 印出計算結果 print(mysum(1, 2, 3)) print(mysum(1.1, 2.2, 3.3)) # 檔名: type_demo2.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
第 2 行,用關鍵字 type 將 Number 設定為 int 或 float ,也就是數字的聯集型態 Union
1 2 | # 設定 Number 為整數或浮點數 type Number = int | float |
這樣在底下定義 mysum() 函數 (function) 就使用 Number 標記
4 5 6 7 8 9 | # 計算總和的函數 def mysum(*p: Number) -> Number: result = 0 for i in p: result += i return result |
單元 44 - 函數與 return 陳述會介紹如何定義函數,至於型態標記 (type hint) 在單元 9 - 型態標記介紹。
其實這個程式有沒有標記都不受影響,因為 Python 本來就允許變數 (variable) 包括參數 (parameter) 自動取得型態,但是型態標記的好處是程式的可讀性更高,相對讓程式碼也更容易維護。
關鍵字 type 也支援將串列 (list) 或序對 (tuple) 設定型態,像是
| type Point = tuple[float, float] |
單元 38 - 序對與 Range會介紹如何使用序對,單元 39 - 串列會介紹如何使用串列。
同樣的,以上語法在 Python 3.12 可以順利執行,但作用僅為型態標記,至於型態檢查是靠第三方工具來做,也就是說,型態檢查的第三方工具必須支援到 Python 3.12 才能對該語法進行檢查。
參考資料
- https://docs.python.org/3/reference/simple_stmts.html#the-type-statement
- https://typing.readthedocs.io/en/latest/spec/aliases.html
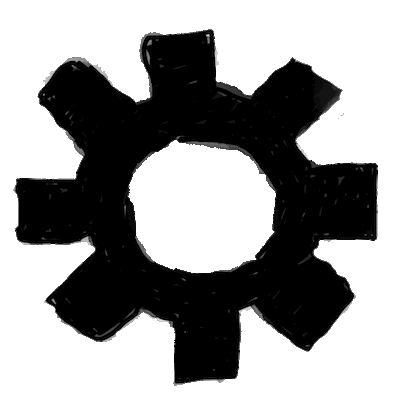