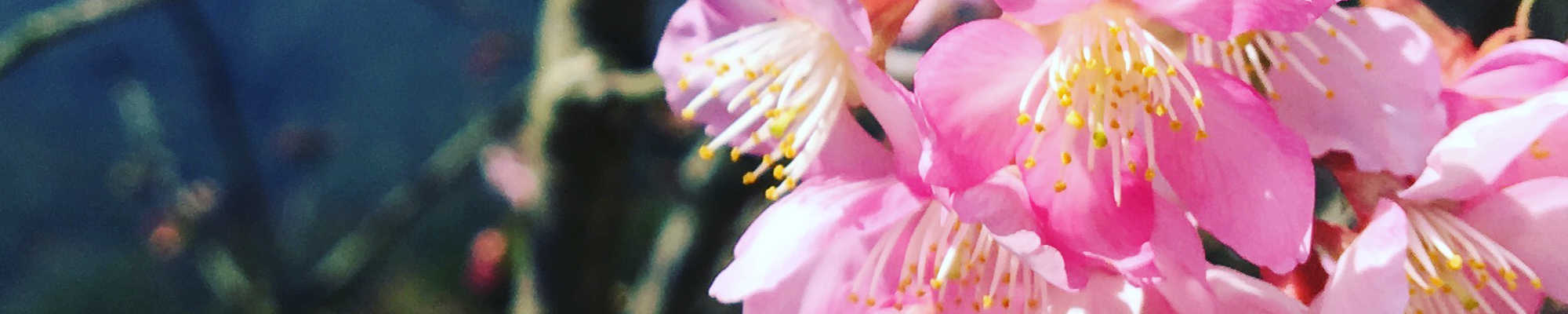
Python 簡易手冊
單元 21 - match 陳述
match 陳述 (statement) 是 Python 3.10 之後新增功能,這是對指定的數值 (value) 進行處理,寫法如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | # 對指定常數做處理 match 3: # 對整數 1 做處理 case 1: print(1) # 對整數 2 做處理 case 2: print(2) # 對以上皆非的情況做處理 case _: print(0) # 檔名: match_demo.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
第 3 行,關鍵字 (keyword) match 後面空一格,然後是要處理的指定數值,這裡是直接用整數 3 ,最後要加上冒號
1 2 | # 對指定常數做處理 match 3: |
一般是取得指定數值放進變數 (variable) 中,然後 match 檢測變數,這裡是示範 match 的用法,所以直接用整數的字面常數 (literal) 。
下面每一個 case 都是針對不同數值進行處理,注意 case 之後也是空一格接上指定數值,最後一樣加上冒號
3 4 5 6 7 8 9 10 11 | # 對整數 1 做處理 case 1: print(1) # 對整數 2 做處理 case 2: print(2) # 對以上皆非的情況做處理 case _: print(0) |
最底下的 case 接的是底線,這裡的底線表示以上皆非,也就是沒有配對到以上的任何 case ,就會自動執行底線的部分,此例執行結果如下
> python match_demo.py |
0 |
> |
match 及 case 如果一次要配對多個數值,就要使用序對 (tuple) 型態,單元 38 - 序對與 Range會詳細介紹如何定義序對。
如果有不同數值要做相同處理,可以在 case 利用 | 運算子提供「或」運算,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | # 對指定常數做處理 match 3: # 對整數 1 或 2 做處理 case 1 | 2: print(1) # 對整數 3 或 4 做處理 case 3 | 4: print(3) # 對以上皆非的情況做處理 case _: print(0) # 檔名: match_demo2.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
注意這裡只能用 | ,不能用 or 。
這樣就變成處理 1 或 2 、 3 或 4 與以上皆非等三種處理方式,此例執行結果如下
> python match_demo2.py |
3 |
> |
每個 case 的指定數值可以再接上 if 判斷條件是否為真,例如
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | # 設定串列資料 fruit = ["apple", "banana", "orange"] # 對指定常數做處理 match "apple": # 檢測不在 fruit 中的情況 case f if f not in fruit: print(1) # 檢測在 fruit 中的情況 case f if f in fruit: print(2) # 對以上皆非的情況做處理 case _: print(0) # 檔名: match_demo3.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
關鍵字 if 主要用作依條件 (condition) 選擇,單元 20 - if 陳述會介紹 if 陳述,單元 15 - 條件運算式介紹條件運算式 (conditional expression) 。
第 2 行先建立串列 (list) 變數 fruit ,在 fruit 中儲存一些水果的英文名稱
1 2 | # 設定串列資料 fruit = ["apple", "banana", "orange"] |
match 之後檢測的是 "apple" ,底下第一個 case 是判斷 "apple" 不在 fruit 中的情況
6 7 8 | # 檢測不在 fruit 中的情況 case f if f not in fruit: print(1) |
這裡的變數 f 代表的是 "apple" ,也是指後面 if 之後的控制變數 (control variable) ,這一行 case 會依序走訪 fruit ,然後確認 "apple" 不是 fruit 的元素,只有當 "apple" 不是 fruit 的元素,這個 case 才會成立,進而執行底下縮排的程式碼。
單元 19 - in 運算子詳細介紹 in 運算子 (operator) 的用法。
由於 "apple" 的確在 fruit ,所以第二個 case 會成立
9 10 11 | # 檢測在 fruit 中的情況 case f if f in fruit: print(2) |
注意以上兩個控制變數的名稱一樣,但是後面的判斷條件不一樣,此例執行結果如下
> python match_demo3.py |
2 |
> |
參考資料
- https://docs.python.org/3/reference/compound_stmts.html#the-match-statement
- https://docs.python.org/3/tutorial/controlflow.html#match-statements
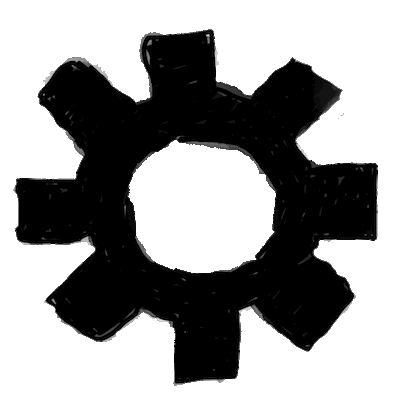