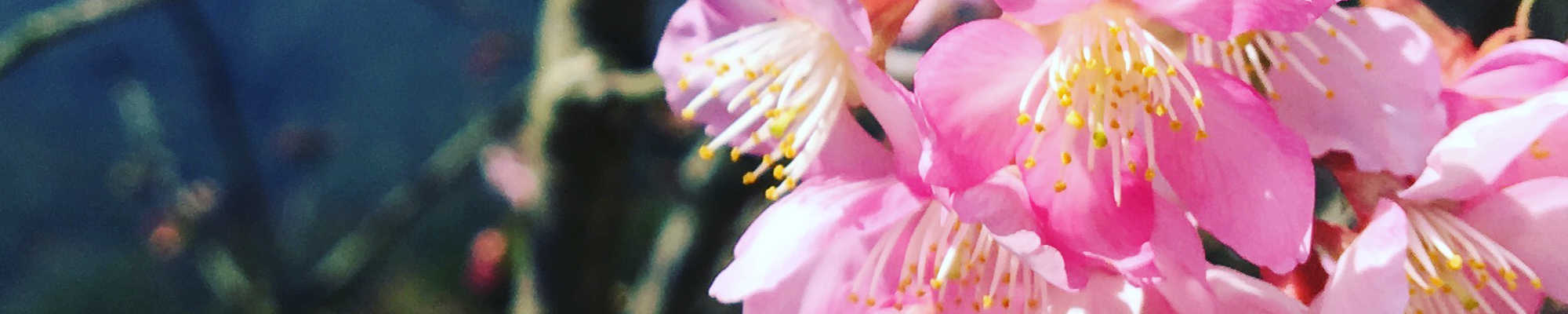
Python 簡易手冊
單元 16 - 位元運算子
資料 (data) 是以二進位數字的方式儲存在電腦中,二進位數字可以用逐位元的方式進行計算, Python 有以下的位元計算子 (bitwise operator)
種類 | 運算子 |
---|---|
非 | ~ |
且 | & |
或 | | |
互斥或 | ^ |
向左位移 | << |
向右位移 | >> |
舉例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | # 設定初始資料 a = 3 print(bin(a)) b = 10 print(bin(b)) # 二進位非運算 print(bin(~a)) # 二進位且運算 print(bin(a & b)) # 二進位或運算 print(bin(a | b)) # 二進位互斥或運算 print(bin(a ^ b)) # 二進位向左位移 print(bin(a << 2)) # 二進位向右位移 print(bin(a >> 2)) # 檔名: bitwise_demo.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 3 月 |
內建函數 (buile-in function) bin() 會回傳引數 (argument) 的二進位數字字串 (string) ,因此像是第 3 行會印出變數 (variable) a 的二進位數字形式
3 | print(bin(a)) |
底下依次示範位元運算子,注意到第 20 行,向左位移的意思是二進位中所有位數往左移動,右側補 0 ,因此向左移動 2 個位元,等同乘上 2 的 2 次方
19 20 | # 二進位向左位移 print(bin(a << 2)) |
向右位移是反過來,所有位數向右移動,如果移動等於或大於該資料的位元數,那會得到 0 ,例如第 23 行的效果
22 23 | # 二進位向右位移 print(bin(a >> 2)) |
此例執行結果如下
> python bitwise_demo.py |
0b11 0b1010 -0b100 0b10 0b1011 0b1001 0b1100 0b0 |
> |
位元運算子可以和指派運算子 (assignment operator) 連在一起用,指派運算子在單元 14 - 指派陳述與指派運算式介紹。
參考資料
- https://docs.python.org/3/reference/expressions.html#binary-arithmetic-operations
- https://docs.python.org/3/reference/expressions.html#shifting-operations
- https://docs.python.org/3/reference/expressions.html#binary-bitwise-operations
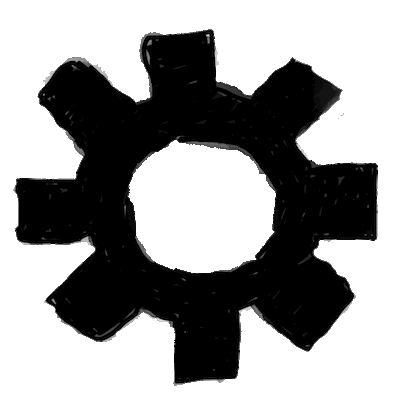