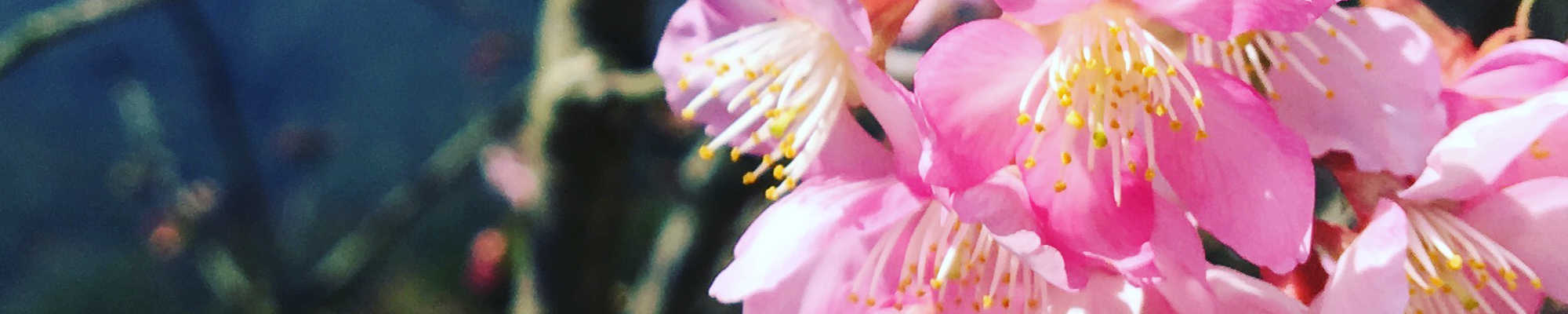
Python 簡易手冊
單元 4 - 文件字串
當開發軟體 (software) 到一定規模之後,往往需要編寫軟體技術文件 (software documentation) 作為輔助,技術文件主要是程式相關定義的說明或教學,因為開發工作往往不是只有自己一個人做,技術文件有助於在移交工作後,讓團隊其他成員理解程式碼 (code) 功能。
Python 利用文件字串 (documentation string) 來寫技術文件,這是直接用三引號字串 (triple-quoted string) 直接定義在程式碼中,然後可以用內建屬性 (attribute) __doc__ 存取,舉簡單例子如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | # 從標準程式庫引入 random import random # 印出 random 的文件字串 print(random.__doc__) # 印出分隔線 print("~~~~~~") # 印出 randint() 的文件字串 print(random.randint.__doc__) # 檔名: doc_demo.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 2 月 |
第 2 行先引入標準程式庫 (standard library) 中的 random 程式庫
1 2 | # 從標準程式庫引入 random import random |
第 5 行印出 random 的文件字串
4 5 | # 印出 random 的文件字串 print(random.__doc__) |
然後第 11 行印出 random 中 randint() 函數 (function) 的文件字串
10 11 | # 印出 randint() 的文件字串 print(random.randint.__doc__) |
簡單說,這個例子先印出 random 的文件字串,然後印出分隔線,最後印出 randint() 的文件字串,執行結果如下
> python doc_demo.py |
Random variable generators. bytes ----- uniform bytes (values between 0 and 255) integers -------- uniform within range sequences --------- pick random element pick random sample pick weighted random sample generate random permutation distributions on the real line: ------------------------------ uniform triangular normal (Gaussian) lognormal negative exponential gamma beta pareto Weibull distributions on the circle (angles 0 to 2pi) --------------------------------------------- circular uniform von Mises discrete distributions ---------------------- binomial General notes on the underlying Mersenne Twister core generator: * The period is 2**19937-1. * It is one of the most extensively tested generators in existence. * The random() method is implemented in C, executes in a single Python step, and is, therefore, threadsafe. ~~~~~~ Return random integer in range [a, b], including both end points. |
> |
由上可以看到 random 的文件字串有很多行,而 randint() 的文件字串只有一行,基本上由於 random 是程式庫,也就是模組 (module) ,裡頭包含很多定義,所以 random 的文件字串就是說明這個模組的功能。
模組是 Python 的程式檔案,凡是副檔名 .py 的 Python 程式檔案都是模組,單元 68 - 模組與 import 陳述會進一步介紹模組與 import 陳述。
原則上文件字串的第一行是簡單扼要地說明功能,其下如有需要,像是提供簡單的使用範例才會有更多的行數,而 randint() 的文件字串僅有說明,事實上 randint() 就是需要提供兩個整數引數 (argument) 才能成功回傳兩個引數之間的整數,所以雖然只有一行也直接說明用法。
定義文件字串使用三引號字串,基本上前後連續三個單引號或雙引號都是三引號字串,但是單引號及雙引號不能在連續三引號中混用,以下例子示範在模組、函數、類別 (class) 及方法 (method) 中定義文件字串
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | """這是模組文件字串 僅作示範用 第三行""" # 定義函數 def do_something(): """這是函數文件字串 僅作示範用 第三行""" pass # 定義類別 class ClassDemo: """這是類別文件字串 僅作示範用 第三行""" # 定義方法 def do_something(): """這是方法文件字串 僅作示範用 第三行""" pass # 印出上述定義的文件字串 print(__doc__) print(do_something.__doc__) print(ClassDemo.__doc__) print(ClassDemo.do_something.__doc__) # 檔名: doc_demo2.py # 說明: 《Python簡易手冊》的範例 # 網址: http://kaiching.org # 作者: Kaiching Chang # 時間: 2024 年 2 月 |
這個例子主要示範定義文件字串的位置,模組文件字串放在檔案開頭,如第 1 行到第 3 行
1 2 3 | """這是模組文件字串 僅作示範用 第三行""" |
函數文件字串放在關鍵字 (keyword) def 的下一行,如第 7 行到第 9 行
5 6 7 8 9 10 | # 定義函數 def do_something(): """這是函數文件字串 僅作示範用 第三行""" pass |
上面關鍵字 pass 表示什麼都不做,單元 49 - pass 陳述與省略符號 ...會詳細介紹 pass 的用法。
類別文件字串放在關鍵字 class 的下一行,如第 14 行到第 16 行
12 13 14 15 16 | # 定義類別 class ClassDemo: """這是類別文件字串 僅作示範用 第三行""" |
方法文件字串一樣放在關鍵字 def 的下一行,如第 20 行到第 22 行
18 19 20 21 22 23 | # 定義方法 def do_something(): """這是方法文件字串 僅作示範用 第三行""" pass |
底下執行部分直接印出這四組文件字串,執行結果如下
> python doc_demo2.py |
這是模組文件字串 僅作示範用 第三行 這是函數文件字串 僅作示範用 第三行 這是類別文件字串 僅作示範用 第三行 這是方法文件字串 僅作示範用 第三行 |
> |
文件字串跟註解 (comment) 類似,屬於程式設計師額外在程式碼中加入的說明部分,雖說沒有強制規定撰寫內容或格式,不過這還是要以開發團隊或專案為主,也就是維持團隊或專案的一貫風格。
參考資料
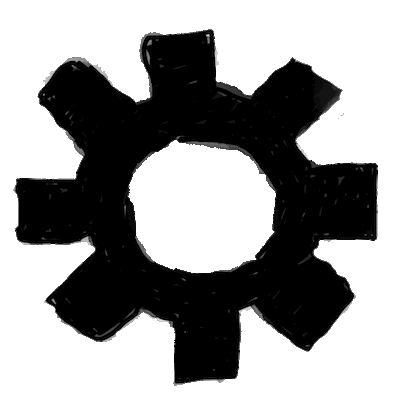